Java Program to smallest among three numbers
- Home
- Find elements
- Java Program to smallest among three numbers
- On
- By
- 0 Comment
- Categories: Find elements
Java Program to smallest among three numbers
Java Program to smallest among three numbers
In this tutorial, we discuss Java Program to the smallest among three numbers
In this program, we will discuss a simple concept of the program to find the smallest number among three numbers in the Java programming language.
In this topic, we learn how to find the smallest number from given three numbers using if statements.
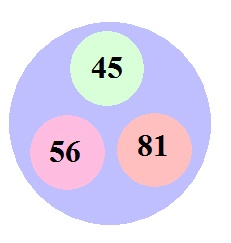
Find smallest among integer numbers
Program 1
import java.util.Scanner; class Small_Three1{ public static void main (String args[]){ Scanner scan=new Scanner(System.in); System.out.print("Enter the first number: "); int num1=scan.nextInt();//get input from user for num1 System.out.print("Enter the second number: "); int num2=scan.nextInt();//get input from user for num2 System.out.print("Enter the third number: "); int num3=scan.nextInt();//get input from user for num3 if(num1<=num2 && num1<=num3){ System.out.println("\n The Smallest number is: "+num1); } if(num2<=num1 && num2<=num3){ System.out.println("\n The Smallest number is: "+num2); } if(num3<=num1 && num3<=num2){ System.out.println("\n The Smallest number is: "+num3); } } }
When the above code is compiled and executed, it produces the following results
Enter the first number: 56 Enter the second number: 23 Enter the tird number: 87 The Smallest number is 23
Find smallest among float numbers
Program 2
In this topic, we learn how to find the smallest number from given three float numbers using if statements.
import java.util.Scanner; class Float_Small{ public static void main (String args[]){ Scanner scan=new Scanner(System.in); System.out.print("Enter the first number: "); float num1=scan.nextFloat();//get input from user for num1 System.out.print("Enter the second number: "); float num2=scan.nextFloat();//get input from user for num2 System.out.print("Enter the third number: "); float num3=scan.nextFloat();//get input from user for num3 if(num1<=num2 && num1<=num3){ System.out.println("\n The Smallest number is "+num1); } if(num2<=num1 && num2<=num3){ System.out.println("\n The Smallest number is "+num2); } if(num3<=num1 && num3<=num2){ System.out.println("\n The Smallest number is "+num3); } } }
When the above code is compiled and executed, it produces the following results
Enter the first number: 5.7 Enter the second number:2.3 Enter the third number: 8.7 The Smallest number is 2.3
Suggested for you
if statements in Java language
Nested if statements in Java language
Class and main method in Java language
Similar post
C code to smallest among three numbers
C++ code to smallest among three numbers
Python code to smallest among three numbers
Java code to find smallest of three numbers using method
C code to smallest among three numbers using function
C++ code to smallest among three numbers using function
Python code to smallest among three numbers using function
Java code to find smallest of three numbers using ternary operator
C code to smallest among three numbers using ternary operator
C++ code to smallest among three numbers using ternary operator