Java Example to subtract two integer using betwise operator
- Home
- Calculations
- Java Example to subtract two integer using betwise operator
- On
- By
- 0 Comment
- Categories: Calculations, subtraction
Java Example to subtract two integer using betwise operator
Java Example to subtract two integer using Bitwise operator
In this article, we will discuss the concept of the Java Example to subtract two integer using Bitwise operator
In this post, we are going to learn how to write a program to find the subtraction of two numbers using Bitwise operator in Java programming language
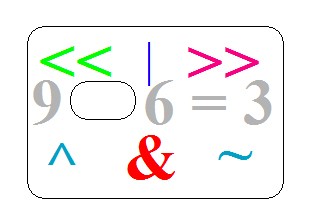
Code to find the subtraction of two numbers
Subtract two integer using Bitwise operator
The program allow the user to enter two integers and then calculates subtraction of given numbers using Bitwise operator
Program 1
//subtract two integer numbers using bitwise in Java import java.util.*; public class SubtractNumBitwise{ public static void main(String args[]){ int x,y; Scanner sc=new Scanner(System.in); //scanner class for input System.out.println("Please Enter the first integer number "); int num1=sc.nextInt(); System.out.println("Please Enter the second integer number "); int num2=sc.nextInt(); //ask input from the user and store the input value to variables x=num1;//Assign the value of num1 to variable x y=num2;//Assign the value of num2 to variable y while(num2!=0){ //Calculate the subtraction using while loop int brw =(~num1)&num2; num1=num1^num2; num2=brw<<1; } System.out.println("Subtraction of two numbers "+x+" and "+y+" is: "+num1); //display result on the screen } }
When the above code is executed, it produces the following result
Case 1
Please Enter the first integer number 250 Please Enter the second integer number 120 Subtraction of two numbers 250 and 120 is: 130
Case 2
Please Enter the first integer number 250 Please Enter the second integer number 500 Subtraction of two numbers 250 and 500 is: -250
Subtract two integer using Bitwise operator with function
Program 2
The program allow the user to enter two integers and then calculates subtraction of given numbers using Bitwise operator
//subtract two integer numbers using bitwise in Java import java.util.*; public class SubtractNumBitwiseMethod{ public static void main(String args[]){ int x,y; Scanner sc=new Scanner(System.in); //scanner class for input System.out.println("Please Enter the first integer number "); int num1=sc.nextInt(); System.out.println("Please Enter the second integer number "); int num2=sc.nextInt(); x=num1;//Assign the value of num1 to variable x y=num2;//Assign the value of num2 to variable y subNum(num1,num2);//calling the function } static void subNum(int num1,int num2){ //method definition while(num2!=0){ int brw =(~num1)&num2; num1=num1^num2; num2=brw<<1; } System.out.println("Subtraction of two numbers is: "+num1); //display result on the screen } }
When the above code is executed, it produces the following result
Case 1
Please Enter the first integer number 2000 Please Enter the second integer number 1300 Subtraction of two numbers is: 700
Case 2
Please Enter the first integer number 1300 Please Enter the second integer number 2000 Subtraction of two numbers is: -700
Suggested for you
Operator in Java language
Data type and variable in Java language
Class and main method in Java language
if statements in Java language
Similar post
Subtract two numbers in Java language
Subtract two numbers using method in Java language
Subtract two numbers using recursion in Java language
Find sum of two numbers in Java language
Find sum of two numbers using method in Java language
Find sum of two numbers in Java using recursion
Find sum of two numbers in Java without Arithmetic operator
Find sum of natural numbers in Java language
Find sum of natural numbers in Java language using recursion