Cpp program to check whether a number is even or odd
- Home
- Calculations
- Cpp program to check whether a number is even or odd
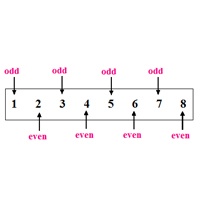
- On
- By
- 0 Comment
- Categories: Calculations, Check value
Cpp program to check whether a number is even or odd
Cpp program to check whether a number is even or odd
In this tutorial, we will discuss the Cpp program to check whether a number is even or odd
In this program, we are going to learn about how to find odd or even number from given number using if else statements in C++ programming language
What is Even or Odd
When the number is divided by 2 and the balance becomes zero. the above number is called as the even number – eg 2,4,6,8,10
and on the other sides when it is divided by 2 and balance becomes 1 there are called odd numbers or uneven numbers
Once This program received the number it will check the given number either odd or even.
After the program received input from the user, it is stored in the variable of num. then program divides the value of num into 2 and displays the output
Using modular operator
Program 1
#include <iostream> #include <conio.h> using namespace std; int main() { int num; cout << "Enter a integer number: " << endl; cin>>num; if(num%2==0) cout<<num<<"is an even"; else cout<<num<<"is an odd"; getch(); return 0; }
When the above code is executed, it produces the following results
case 1
Enter an integer value: 44 44 is an even
case 2
Enter an integer value: 65 65 is an odd
when we use the modular operator, if it is the remainder appears as 0, it is called even number and if the remainder displays the balance as one, it is called as odd or uneven number
Using ternary operator
Program 2
#include <iostream> #include <conio.h> using namespace std; int main() { int num; cout << "Enter an integer number: " << endl; cin>>num; (num%2==0)? cout <<num<<" is an even number":cout<<num<<" is an odd number"; getch(); return 0; }
When the above code is executed, it produces the following results
case 1
Enter an integer value: 78 78 is an even number
case 2
Enter an integer value: 99 99 is an odd number
Using Bitwise operator
Program 3
#include <iostream> #include <conio.h> using namespace std; int main() { int num; cout << "Enter the integer number" << endl; cin>>num; if(num & 1) cout<<num<<"is an odd number"; else cout<<num<<"is an even number"; getch(); return 0; }
When the above code is executed, it produces the following results
case 1
Enter an integer value: 43 43 is an odd number
case 2
Enter an integer value: 68 68 is an even number
Using divisional operators
Program 4
#include <iostream> #include <conio.h> using namespace std; int main() { int num; cout << "Enter the integer number" << endl; cin>>num; if((num /2)*2==num) cout<<num<<"is an even number"; else cout<<num<<"is an odd number"; getch(); return 0; }
When the above code is executed, it produces the following results
case 1
Enter an integer value: 88 88 is an even number
case 2
Enter an integer value: 47 47 is an odd number
Program 5
Using shift operators
#include <iostream> #include <conio.h> using namespace std; int main() { int num; cout << "Enter the number" << endl; cin>>num; if(((num>>1)<<1)==num ){ cout<<num<<"is even "; } else{ cout<<num<<"is odd"; } getch(); return 0; }
When the above code is executed, it produces the following results
case 1
Enter an integer value: 124 88 is even
case 2
Enter an integer value: 151 151 is odd
Similar post
C++ program to find a number is even or odd using the function
C++ program to separate Odd and Even numbers from an array
C++ program to display all even and odd numbers from 1 to n
C++ program calculate Average of odd and even numbers in an array
C++ program to calculate the sum of odd and even numbers
C++ program to find whether a number is even or odd
Java program to find a number is even or odd using the method
Java program to separate Odd and Even numbers from an array
Java program to display all even and odd numbers from 1 to n
Java program display odd and even numbers without if statements
Java program to calculate the sum of odd and even numbers
Java program to find whether a number is even or odd
Suggested for you
Data type in C++ language