Triangle Hollow Pattern using nested while loop in Cpp
- Home
- Floyd's triangle
- Triangle Hollow Pattern using nested while loop in Cpp
- On
- By
- 0 Comment
- Categories: Floyd's triangle, star pattern
Triangle Hollow Pattern using nested while loop in Cpp
Triangle Hollow Pattern using nested while loop in Cpp
In this tutorial, we will discuss Triangle Hollow Pattern using nested while loop in Cpp
In this program, we are going to learn about how to display Hollow Tringle star pattern using nested while loop in C++ programming language
Here, we display an Hollow triangle Pattern program with coding using nested while loop and also program get input from the user using Cin function in C++ programming language
The user can provide numbers as they wish and get the Hollow Triangle pattern according to their input.
Floyd’s triangle star pattern 1
Program 1
#include <iostream> #include <conio.h> using namespace std; int main() { int rows; cout<<"Enter the number of rows" << endl; cin>>rows; int i,j; i=1; while(i<=rows){ j=1; while(j<=i){ if(j==1 || j==i ||i==rows ) cout<<"*"; else cout<<" "; j++;; } i++; cout <<"\n";//Move to the next line for print } getch(); return 0; }
When the above code is executed, it produces the following results
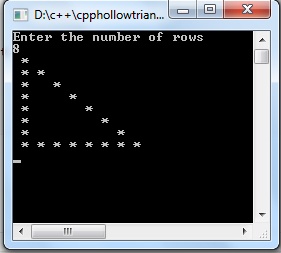
Floyd’s triangle star pattern 2
Program 2
#include <iostream> #include <conio.h> using namespace std; int main() { int rows; cout<<"Enter the number of rows" << endl; cin>>rows; int i,j; i=1; while(i<=rows){ j=i; while(j<rows){ cout<<" "; j++; } j=1; while(j<=i){ if(j==i || j==1 ||i==rows ) cout<<"*"; else cout<<" "; j++;; } i++; cout<<"\n";//Move to the next line for print } getch(); return 0; }
When the above code is executed, it produces the following results
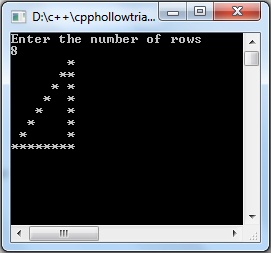
Floyd’s triangle star pattern 3
Program 3
#include <iostream> #include <conio.h> using namespace std; int main() { int rows; cout<<"Enter the number of rows" << endl; cin>>rows; int i,j; i=1; while(i<=rows){ j=1; while(j<=rows){ if(j==i || j==rows ||i==1 ) cout<<"*"; else cout<<" "; j++;; } i++; cout<<"\n";//Move to the next line for print } getch(); return 0; }
When the above code is executed, it produces the following results
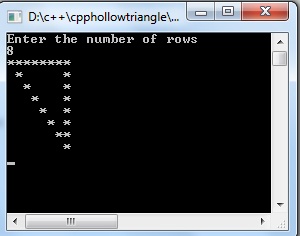
Floyd’s triangle star pattern 4
Program 4
#include <iostream> #include <conio.h> using namespace std; int main() { int rows; cout<<"Enter the number of rows" << endl; cin>>rows; int i,j; i=1; while(i<=rows){ j=i; while(j<=rows){ if( i==1|| j==i || j==rows) cout<<"*"; else cout<<" "; j++;; } i++; cout<<"\n";//Move to the next line for print } getch(); return 0; }
When the above code is executed, it produces the following results
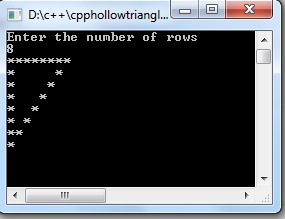
Suggested for you
Data type in C++ language
Nested while loop in C++ language