Take Array input in Java language
Take Array input in Java language
In this tutorial, we will discuss the concept of Take Array input in Java language
In this topic, we are going to learn how to input the integer array elements in Java programming language using for, while and do-while loops.
In this blog, We have already discuss that “What is an array“, type of arrays and how to access it(one dim, two dim and three dim arrays)
Arrays are the special type of variables to store Multiple type of values(int, string,char, etc)under the same name in the continuous memory location. we can be easily accessed using the indices(indexes)
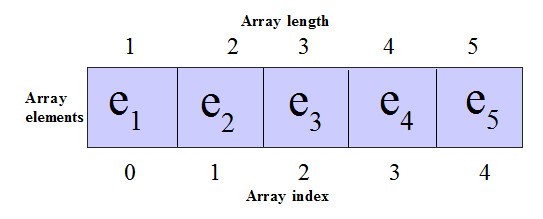
Here,
- e1,e2,e3,e4 and e5 represent the integer elements of the array
- 0,1,2,3 and 4 represent the index of the array which is used to access the array elements
- 1,2,3,4 and 5 represent the length of the array
Code to input array elements in Java
Program to read elements in array using for loop
In this program, we are briefing how to input integer elements of an array using for loop in Java language
Program 1
import java.util.Scanner; public class TakeArrayInputfor{ public static void main(String args[]){ //scanner class object to read input Scanner sc=new Scanner(System.in); //Declaring and creating array int[] arr=new int[5]; //Display dfault value before initialize System.out.println("Default value of integer array: "); for(int i=0; i<arr.length; i++){ System.out.println(arr[i]+"\t"); } System.out.println(""); //initializing the array System.out.println("******Initializing array******"); System.out.println("Enter "+arr.length+" integer values"); for(int i=0; i<arr.length; i++){ arr[i]=sc.nextInt(); } //displaying the array System.out.println("\n******displaying array elements******"); System.out.println("Entered array elements are"); for(int i=0; i<arr.length; i++){ System.out.println(arr[i]+"\t"); } } }
When the above code is executed, it produces the following result
Default value of integer array: 0 0 0 0 0 *****initializing array****** Enter 5 integer value 35 58 79 95 70 *****displaying array elements****** Entered array elements are: 35 58 79 95 70
Program to read elements in array using while loop
In this program, we are briefing how to input integer elements of an array using while loop in Java language
Program 2
import java.util.Scanner; public class TakeArrayInputwhile{ public static void main(String args[]){ //scanner class object to read input Scanner sc=new Scanner(System.in); //Declaring and creating array int[] arr=new int[5]; //Display dfault value before initialize System.out.println("Default value of integer array: "); int i=0; while(i<arr.length){ System.out.println(arr[i]+"\t"); i++; } System.out.println(""); //initializing the array System.out.println("******Initializing array******"); System.out.println("Enter "+arr.length+" integer values"); i=0; while(i<arr.length){ arr[i]=sc.nextInt(); i++; } //displaying the array System.out.println("\n******displaying array elements******"); System.out.println("Entered array elements are"); i=0; while(i<arr.length){ System.out.println(arr[i]+"\t"); i++; } } }
When the above code is executed, it produces the following result
Default value of integer array: 0 0 0 0 0 *****initializing array****** Enter 5 integer value 356 876 487 890 987 *****displaying array elements****** Entered array elements are: 356 876 487 890 987
Program to read elements in array using do-while loop
In this program, we are briefing how to input integer elements of an array using do-while loop in Java language
Program 3
import java.util.Scanner; public class TakeArrayInputDowhile{ public static void main(String args[]){ //scanner class object to read input Scanner sc=new Scanner(System.in); //Declaring and creating array int[] arr=new int[5]; //Display dfault value before initialize System.out.println("Default value of integer array: "); int i=0; do{ System.out.println(arr[i]+"\t"); i++; }while(i<arr.length); System.out.println(""); //initializing the array System.out.println("******Initializing array******"); System.out.println("Enter "+arr.length+" integer values"); i=0; do{ arr[i]=sc.nextInt(); i++; }while(i<arr.length); //displaying the array System.out.println("\n******displaying array elements******"); System.out.println("Entered array elements are"); i=0; do{ System.out.println(arr[i]+"\t"); i++; }while(i<arr.length); } }
When the above code is executed, it produces the following result
Default value of integer array: 0 0 0 0 0 *****initializing array****** Enter 5 integer value 456 78 999 654 567 *****displaying array elements****** Entered array elements are: 456 78 999 654 567
Similar post
Code to read and print integers of an array in Java
Code to read and print strings of an array in Java
Code to read and print characters of an array in Java
Code to read and print integers of an array in C++
Code to read and print strings of an array in C++
Code to read and print characters of an array in C++
Code to read and print integers of an array in C
Code to read and print strings of an array in C
Suggested for you
One dimensional array in C language
One dimensional array in C++ language
Two dimension array in Java language
Two dimension array in C language
Two dimension array in C++ language
Three dimension array in Java language
Three dimension array in C language
Three dimension array in C++ language
Do-while loop in Java language
Enhanced for loop in Java language
Data type and variable in Java language