- On
- By
- 0 Comment
- Categories: Array, Data types
Single dimension Array of C language
Single dimension Array of C language
In this tutorial, we will discuss this in Single dimension Array in C language
An array is a collection of data structures that consist of fixed(cannot change array length) a number of values of the same type such as int, char, float etc… If the data can be viewed and entered entirely in one dimension, it is called Single dimension Array in C programming language.
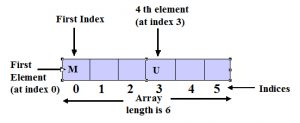
Always, Array index starts from 0, not 1
Array index ends in n-1(n is equal to array size or array length)
Array length(size) starts from 1 and ends in n(n is array length)
Array declaration, creation, initiation
How to declare of an array
Syntax
data_type array_name[array_size];
For example
double salary[40];
int marks[20];
char name[50];
Here, we declared a one dimension array, an array name is salary and array size is 40. It means, it can allocate 40 memory space to store double value.
the array size and type cannot be changed after this declaration.
Declare number of n element array
Syntax
data_type array_name[n];
Example
int age[n];
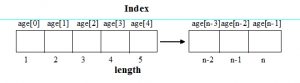
Here, we declared an int type array named age with size equal to n. It means allocating only n(number of) number of memory space to save only int data type(similar data type).
Note – The size and type can not be changed after its declaration
How is C array represented in memory
int marks[6];
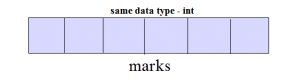
When we declare an array, memory allocates the fixed size of space according to the array size.
In the above example, we declared an array size of 6 as an integer. So, memory allocates six places for array data in memory.
How to initialize an array in C language
Method 1
initialized to every index
number[o]=34; //initialized number[o] by 34
number[1]=444; //initialized number[1] by 444
number[2]=789; //initialized number[2] by 789
number[3]=340; // initialized number[3] by 340
number[4]=241; // initialized number[4] by 241
Method 2
int marks[6]={67,76,45,65,87,83}
When we initialized the array in C, the size of the array is given square brackets[] and the same number of the elements are between the curly brackets {} – Array size can not be changed in programming
Method 3
int age[]={12,32,43,35,54,65}
The size of the array is automatically allocated based on the value of the number in curly brackets
Here, we assigned 6 value in Array, So array length is 6
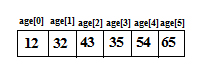
Value_1 12 is placing index age[0]
Value_2 32 is placing index age[1]
Value_3 43 is placing index age[2]
Value_4 35 is placing index age[3]
Value_5 54 is placing index age[4]
Value_6 65 is placing index age[5]
Accessing Array elements
Insert the element in Array of various way
Normal insertion
marks[4]=24; //insert 24 to 4th index of array - 5th element scanf("%d",&marks[3]); //ask element from the user to 4th element(index 3 ) for insertion scanf("%d",&marks[i]); //ask input from the user (i+1) th element(index i) for insertion
Display element from the array
//Normal retrieving printf("%d",marks[2]); //display or retrieve third(index [2]) element of array printf("%d",marks[i]); //Array length l=i+1 //Retrieving i+1 element of array
Example 1
#include <stdlib.h> int main() { int marks[6];//declaration and creation of array marks[0]=34; //normal initialization to index 0 marks[1]=66; marks[2]=74; marks[3]=84; marks[4]=68; marks[5]=87;//normal initialization to index 5 //displaying elements from array using derectely array index printf("%d\n",marks[0]); printf("%d\n",marks[1]); printf("%d\n",marks[2]); printf("%d\n",marks[3]); printf("%d\n",marks[4]); printf("%d\n",marks[5]); printf("End the program\n"); return 0; }
When the above program is compiled and executed, it produces the following results
34 64 74 84 68 87 End the program
Example 2
#include <stdio.h> #include <stdlib.h> int main() { int marks[]={45,65,35,88,64,32,67}; //Array declaration and initialization in same line printf("the first element of the array : %d\n", marks[0]); printf("the second element of the array : %d\n",marks[1]); printf("the third element of the array : %d\n", marks[2]); printf("the forth element of the array : %d\n", marks[3]); printf("the fifth element of the array : %d\n", marks[4]); printf("the sixth element of the array : %d\n", marks[5]); printf("the seventh element of the array : %d\n", marks[6]); //Display array elements getch(); return 0; }
When the above program is compiled and executed, it produces the following results
The first element of the array: 45 The second element of the array: 65 The third element of the array: 35 The fourth element of the array: 88 The fifth element of the array: 64 The sixth element of the array: 32 Seventh element of the array: 67
Array with for loop
Single dimension array in C language to access the elements using for loop
Insert element using for loop
for(i=0; i<=10; i++) { scanf("%d",&a[i]); }//ask element from the user to array using for loop
Displaying element from the array using for loop
for(i=0; i<=10; i++) { printf("%d",a[i]); }//display element from array using for loop
Program 1
#include <stdio.h> #include <stdlib.h> int main() { int marks[5],i; for(i=0; i<=4; i++){//input using for loop printf("Enter the value marks[%d] :",i); scanf("%d",&marks[i]); } printf("\nOutput here\n\n"); printf("index[4] value is :%d\n",marks[4]); printf("index[3] value is :%d\n",marks[3]); printf("index[2] value is :%d\n",marks[2]); printf("index[1] value is :%d\n",marks[1]); printf("index[0] value is :%d\n",marks[0]); printf("End the program\n"); getch(); return 0; }
When the above program is compiled and executed, it produces the following result
Enter the value marks[0] :45 Enter the value marks[1] :67 Enter the value marks[2] :87 Enter the value marks[3] :89 Enter the value marks[4] :87 Output here index[4] value is :87 index[3] value is :89 index[2] value is :87 index[1] value is :67 index[0] value is :45 End the program
Program 2
#include <stdio.h> #include <stdlib.h> int main() { int Numbers[5],i; for(i=0; i<=4; i++){ printf("Enter the value Numbers[%d] :",i); scanf("%d",&Numbers[i]); } printf("\nOutput here\n"); for(i=0; i<=4; i++){ printf("%d\n",Numbers[i]); } printf("End the program\n"); getch(); return 0; }
When the above program is compiled and executed, it produces the following result
Enter the value Numbers[0] :5 Enter the value Numbers[1] :4 Enter the value Numbers[2] :3 Enter the value Numbers[3] :2 Enter the value Numbers[4] :1 Output here 5 4 3 2 1 End the program
Array with while loop
Single dimension array in C language to access the element using the while loop
iterating an array element from the array using while loop
insert element using the while loop
while(i<=10){ scanf("%d",&array_name[i]; }
display element using while loop
while(i<=10){ printf("%d\n",array_name[i]; }
Program 1
#include <stdio.h> #include <stdlib.h> int main() { const char* arr[]={"Sana","Kuna","Jhon","Nimal","Kaju","Amal"}; //declaring a string array int i=0; while(i<=5){ //display array elements using while loop printf("Array index %d: %s\n",i,arr[i]); i++; } getch(); return 0; }
When the above program is compiled and executed it produces the following result
Array index 0: Sana Array index 1: kuna Array index 2: Jhon Array index 3: Nimal Array index 4: Kaju Array index 5: amal
Program 2
#include <stdio.h> #include <stdlib.h> int main() { int marks[8]; int i=0; printf("Enter element for array\n"); while(i<=7)//get input from user using while loop { scanf("%d",&marks[i]); i++; } printf("your marks here\n"); int j=0; while(j<=7)//Display array elements using while loop { printf("%d\n",marks[j]); j++; } printf("End program\n"); getch(); return 0; }
When the above program is compiled and executed, it produces the following result
Enter elements for array 45 65 36 86 43 58 79 98 your marks here 45 65 36 86 43 58 79 98 End the program
Similar post
Similar post
Single dimension Array in C++ language
Two dimension Array in C++ language
Three dimension Array in C++ language
Single dim- Array in Java language
Two dim- Array in Java language
Three dim- Array in Java language
Single dim- Array in C language
Three dim- Array in C language
Suggested for you
Nested for loop in C++ language
Nested while loop in C++ language