Recursion in C programming language
- Home
- function/method
- Recursion in C programming language
- On
- By
- 0 Comment
- Categories: function/method
Recursion in C programming language
Recursion in C programming language
In this tutorial, we will discuss recursion in C programming language
A function calling itself during its execution. it is called a recursive function. another word, a function calling from the definition of the same function is known as a recursion
Example of recursive function
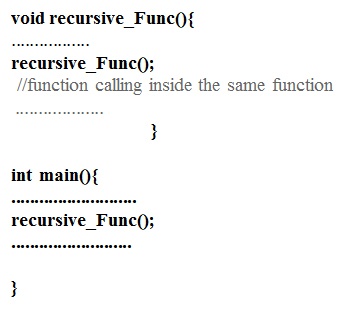
The flow of control of recursive function
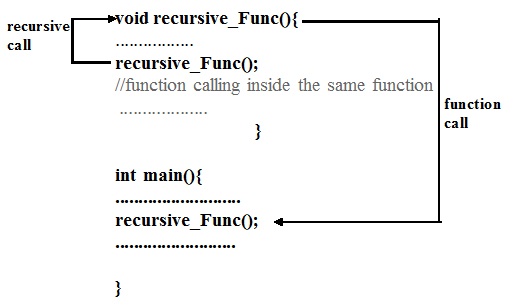
Find factorial using the recursive function
#include <stdio.h> #include <stdlib.h> int find_Factorial(int n);//declare a function to find the factorial. int main() { int num; printf("Enter the number to find factorial: "); scanf("%d",&num); printf("Factorial of %d=%d", num, find_Factorial(num));//call the function inside the main() function getch(); return 0; } //user defined function int find_Factorial(int num) //define the function according to declaration { if(num<1) return 1; else return num*find_Factorial(num-1);//find_Factorial function calls itself }
When the above code is executed, it produces the following results
Enter the number to find factorial: 5 Factorial of 6 = 120
In the above program, first, find_Factorial() function called inside the main () function with passing its argument.
when the value passed to the function, it is stored in the num variable.
Initially, the value of num is 5 to pass as an argument, then the function calls itself, in it the value of num reduce by 1 and it becomes 4 and it passes to beginning “find_Factorial()” function. it continuously reduces by one in every stage until it comes to 0.
when the num becomes less then 1, here, the if condition returns false and flow of control moves to else part for execution.
else part is executed and excludes from the function
Explanation of recursive function to find factorial
Advantages of recursion in C
Easy to understand and the code becomes readable and reduces the number of lines of the program.
This recursion is used to make a complex task easy and also flexible and repeatedly functioning is easier with using nesting iteration
Disadvantages of recursion in C
Tracing and debugging are very difficult
Every recursive lacks a separate memory location, as extra memory is required process becomes very slow
The same programs like this
Suggested for you
scanf() printf() in C language
C++ program to find the power of a number using recursion
C program to find the power of a number using recursion
Java program to find the power of a number using recursion