Python program to find smallest of three numbers
- Home
- Find elements
- Python program to find smallest of three numbers
- On
- By
- 1 Comment
- Categories: Find elements
Python program to find smallest of three numbers
Python program to find the smallest of three numbers
In this tutorial, we will discuss a simple concept of the Python program to find the smallest of three numbers
In this post, we will learn how to find the smallest number among three numbers in the Python programming language.
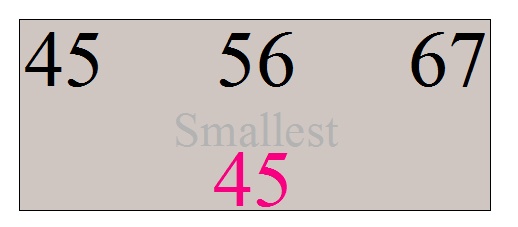
Approaches
- Initially, the program will check if the first number is smaller than the second and third number. If it is true, the printing function will take place and it will be printed
- If the above is false, then it will check if the second number is smaller than the first and third number. it will be printed
- If both the above situation becomes false finally the third number will be printed as it is the smaller number.
Find the smallest number using if elif statements
Program 1
This program allows the user to enter three numbers and compare to select the smallest number using if elif statements
num1=int(input("Enter the first number: ")) #Take input from user for first number num2=int(input("Enter the second number: "))#Take input from user for second number num3=int(input("Enter the third number: ")) #Take input from user for third number if(num1<=num2 and num1<=num3): #Compare the first number with the second and third number print(num1," is the smallest") elif(num2<=num1 and num2<=num3):#Compare the second number with the first and third number print(num2," is the smallest") else: print(num3," is the smallest")
When the above code is executed, it produces the following results
Enter the first number: 45 Enter the second number: 65 Enter the third number: 78 45 is the smallest
Find the smallest number using nested if statements
Program 2
This program allows the user to enter three numbers and compare to select the smallest number using nested if statements
num1=int(input("Enter the first number: ")) num2=int(input("Enter the second number: ")) num3=int(input("Enter the third number: ")) if(num1<=num2): if(num1<=num3): Smallest=num1 else: Smallest=num3 else: if (num2<=num3): Smallest=num2 else: Smallest=num3 #display the smallest number print("Smallest number is", Smallest)
When the above code is executed, it produces the following results
Enter the first number: 57 Enter the second number: 23 Enter the third number: 89 Smallest number is 23
Smiler post
C++ code to find the smallest of three numbers using the function
C program to find the smallest among three numbers using the function
Python code to find the smallest of three numbers using the method
Java code to find the smallest of three numbers using the method
C program to find the largest among three numbers using function
Python code to find the largest of three numbers using the function
C++ code to find the largest of three numbers using the function
Java code to find the largest of three numbers
C code to find the largest of three numbers
C++ code to find the largest of three numbers
Python code to find the largest of three numbers
Java program to find the middle of three number using Method
C code to find the middle of three number using the function
C++ code to find the middle of three number using the function
Python code to find the middle of three number using the function
Java code to find middle of three numbers
C code to find middle of three numbers
C++ code to find middle of three numbers
Python code to find middle of three numbers
Java code to find largest of three numbers in an array
Java code to find smallest of three numbers in an array
C code to find the middle of three number using the function
C++ code to find the middle of three number using the function
Suggested for you
Nested if statement in C++ language
Data type in C++ language
The operator in Python language
If statement in Python language
Data types and Variable in Python
Datatype and variables in Java programming language
If statements in Java language
Nested if statements in the Java language
Nested if statements in C language
1 Comment
Python Ulagam September 30, 2020 at 3:57 pm
Python Program to Find the Smallest Product of Three Integers
Visit: https://pythonulagam.blogspot.com/2020/08/python-small-product-three-integer.html