- On
- By
- 0 Comment
- Categories: Loop, star pattern, While loop
Program to print Rhombus and Hollow Rhombus star Using while loop in C
Program to print Rhombus and Hollow Rhombus star Using while loop in C
In this article, we will discuss the concept of Program to print Rhombus and Hollow Rhombus star pattern Using while loop in C language
In this post, we are going to learn how to use while loop for write a program to print rhombus and hollow rhombus pattern using given symbol in C language.
Display Rhombus patterns Using while loop
Code to print Rhombus pattern using while loop
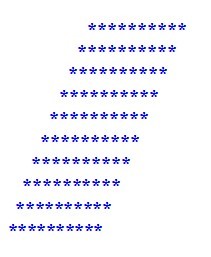
Program 1
This program allows the user to enter the number of rows and symbols then it will display the rhombus pattern using while loop in C programming language
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; char ch; printf("Enter the number of rows\n"); scanf("%d%c",&rows,&ch); printf("Enter the Symbol for pattern\n"); ch=getchar(); printf("\n"); i=1; while(i<=rows){//outer for loop j=1; while(j<=rows-i){//inner for loop printf(" ");//print space j++; } j=1; while(j<=rows){//inner for loop printf("%c",ch);//print character after space j++; } printf("\n");//move to next line i++; } getch(); return 0; }
When the above code is executed, it produces the following result
Code to print Hollow Rhombus pattern using while loop
This program allows the user to enter the number of rows and symbols then it will display the Hollow rhombus pattern using while loop in C programming language
Program 2
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; char ch; printf("Enter the number of rows\n"); scanf("%d%c",&rows,&ch); printf("Enter the Symbol for pattern\n"); ch=getchar(); printf("\n"); i=1; while(i<=rows){//outer for loop j=1; while(j<=rows-i){//inner for loop printf(" ");//print space j++; } j=1; while(j<=rows){//inner for loop if(i==1 || i==rows || j==1 || j==rows){ printf("%c",ch);//print symbol after space } else{ printf(" ");//print space } j++; } printf("\n");//move to next line i++; } getch(); return 0; }
When the above code is executed, it produces the following result
Suggested for you
Nested while loop in C language
Nested Do-while loop in C language
Similar post
Java code to Print Hollow rectangle and square star pattern
C code to Print Hollow rectangle and square star pattern
C++ code to Print Hollow rectangle and square star pattern
Display solid rectangle and square star pattern in Java
Display solid rectangle and square star pattern in C++
Rhombus and hollow rhombus star pattern in C language