- On
- By
- 0 Comment
- Categories: Array, do-while, for loop, Loop, Number pattern, While loop
Program to Generate Pascal’s triangle using 2 D array in Java
Program to Generate Pascal’s triangle using 2 D array in Java
In this tutorial, we will discuss the concept of the Program to Generate Pascal’s triangle pattern using 2 D array in Java
In this topic, we are going to learn how to write a program to print Pascal triangle number patterns using a two dimension Array in the Java programming language
Here, we use for, while, and do-while loops for printing pascal triangle

Display the pascal triangle in Java using loops

Java Code to display pascal triangle using for loop
In this program, the user declares and initializes integer variables, it will display a pascal triangle number pattern using for loop in the Java language according to the rows
Program 1
//Java programto print pascal triangle using 2D Array import java.util.Scanner; //class diclaration class Disp_Pascal_Triangle_2DArr{ public static void main (String args[]){//main method Scanner scan=new Scanner(System.in); //Scanner object for user input System.out.print("Enter the Number of rows: "); //Ask input from the user for row int row=scan.nextInt();//read the input from the user int i=0,j=0,count=row-1;//declare variables int arr[][]=new int[50][50];//declare two D array for(i=0; i<row; i++){ arr[i][i]=arr[i][0]=1; } for(i=0; i<row; i++){ for(j=1; j<i; j++){ arr[i][j]=arr[i-1][j-1]+arr[i-1][j]; } } for(i=0; i<row; i++){ for(j=0; j<count; j++){ System.out.print(" "); } for(j=0; j<=i; j++){ System.out.print(arr[i][j]+" "); } System.out.println(); count--; } } }
When the above code is executed, it produces the following result
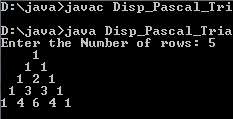
Java Code to display pascal triangle using while loop
In this program, the user declares and initializes integer variables, it will display a pascal triangle number pattern using the while loop in the Java language according to the rows
Program 2
//Java programto print pascal triangle using 2D Array import java.util.Scanner; //class diclaration class Disp_Pascal_Triangle_2DArrWhil{ public static void main (String args[]){//main method Scanner scan=new Scanner(System.in); //Scanner object for user input System.out.print("Enter the Number of rows: "); //Ask input from the user for row int row=scan.nextInt();//read the input from the user int i=0,j=0,count=row-1;//declare variables int arr[][]=new int[50][50];//declare two D array i=0; while(i<row){ arr[i][i]=arr[i][0]=1; i++; } i=0; while(i<row){ j=1; while(j<i){ arr[i][j]=arr[i-1][j-1]+arr[i-1][j]; j++; } i++; } i=0; while(i<row){ j=0; while(j<count){ System.out.print(" "); j++; } j=0; while(j<=i){ System.out.print(arr[i][j]+" "); j++; } System.out.println(); count--; i++; } } }
When the above code is executed, it produces the following result
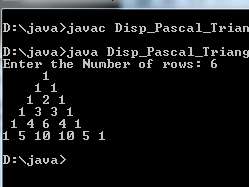
Java Code to display pascal triangle using do-while loop
In this program, the user declares and initializes integer variables, it will display a pascal triangle number pattern using the do-while loop in the Java language according to the rows
Program 3
//Java programto print pascal triangle using 2D Array import java.util.Scanner; //class diclaration class Disp_Pascal_Triangle_2DArrDoWhile{ public static void main (String args[]){//main method Scanner scan=new Scanner(System.in); //Scanner object for user input System.out.print("Enter the Number of rows: "); //Ask input from the user for row int row=scan.nextInt();//read the input from the user int i=0,j=0,count=row-1;//declare variables int arr[][]=new int[50][50];//declare two D array i=0; do{ arr[i][i]=arr[i][0]=1; i++; }while(i<row); i=0; do{ j=1; while(j<i){ arr[i][j]=arr[i-1][j-1]+arr[i-1][j]; j++; } i++; }while(i<row); i=0; do{ j=0; while(j<count){ System.out.print(" "); j++; } j=0; do{ System.out.print(arr[i][j]+" "); j++; }while(j<=i); System.out.println(); count--; i++; }while(i<row); } }
When the above code is executed, it produces the following result

Suggested for you
Data type and variable in Java language
The operator in the Java language
Do-while loop in Java language
Similar post
Java program to print pascal triangle
C program to print pascal triangle
C++ program to print pascal triangle
Java program to print pascal triangle using an array
Java program to print pascal triangle using array using user input
C program to print a pascal triangle using an array
C program to print pascal triangle using array using user input