Program to display combined Pyramid pattern in C using while loop
- Home
- Number pattern
- Program to display combined Pyramid pattern in C using while loop
- On
- By
- 0 Comment
- Categories: Number pattern, pyramid triangle, star pattern
Program to display combined Pyramid pattern in C using while loop
Program to display combined Pyramid pattern in C using while loop
In this tutorial, we will discuss the concept of a C program to display combined Pyramid pattern
In the C programming language, we can use for loop, while loop and do-while loop to display different number (binary, decimal), alphabets or star pattern programs.
In this article, we are going to learn how to Display combined pyramid pattern using while loop in C language
Display combined Pyramid star pattern
Combined Pyramid star pattern 1
Program 1
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: \n"); scanf("%d",&rows); printf("\n"); i=1; while(i<=rows){ //parent while loop j=i; while(j<=rows){ printf(" ");//print space j++; } j=1; while( j<=2*i-1){ printf("*");//print star j++; } j=2*i; while(j<=2*rows-1){ printf(" ");//print space j++; } j=1; while( j<=2*i-1){ printf("*");//print star j++; } printf("\n");//move to next line i++; } getch(); return 0; }
When the above code is executed, it produces the following results
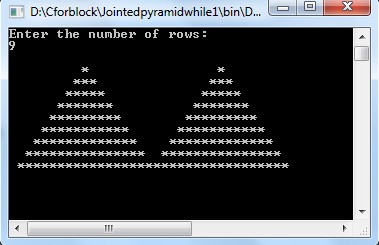
The C program allows the user to enter the number of rows then it displays two combined straight pyramid star pattern according to the number of rows.
Combined Pyramid star pattern 2
Program 2
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: \n"); scanf("%d",&rows); printf("\n"); i=1; while( i<=rows){ j=1; while(j<i){ printf(" "); j++; } j=1; while(j<2*(rows-i+1)){ printf("*"); j++; } j=2; while(j<2*i){ printf(" "); j++; } j=1; while( j<2*(rows-i+1)){ printf("*"); j++; } printf("\n"); i++; } getch(); return 0; }
When the above code is executed, it produces the following results
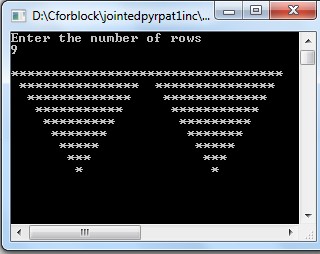
The C program allows the user to enter the number of rows then it displays two combined upside-down pyramid star pattern according to the number of rows.
Display combined Pyramid number pattern
Combined Pyramid number pattern 1
Program 1
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: \n"); scanf("%d",&rows); printf("\n"); i=1; while(i<=rows){ //parent while loop j=i; while(j<=rows){ printf(" ");//print space j++; } j=1; while( j<=2*i-1){ printf("%d",j);//print star j++; } j=2*i; while(j<=2*rows-1){ printf(" ");//print space j++; } j=1; while( j<=2*i-1){ printf("%d",j);//print star j++; } printf("\n");//move to next line i++; } getch(); return 0; }
When the above code is executed, it produces the following results
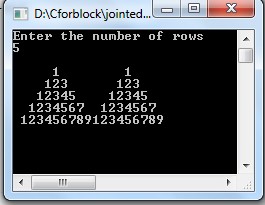
The C program allows the user to enter the number of rows then it displays two combined straight pyramid number pattern according to the number of rows.
Combined Pyramid number pattern 2
Program 2
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: \n"); scanf("%d",&rows); printf("\n"); i=1; while( i<=rows){ j=1; while(j<i){ printf(" ");//print space j++; } j=1; while(j<2*(rows-i+1)){ printf("%d",j);//print number j++; } j=2; while(j<2*i){ printf(" ");//print space j++; } j=1; while( j<2*(rows-i+1)){ printf("%d",j);//print number j++; } printf("\n"); i++; } getch(); return 0; }
When the above code is executed, it produces the following results
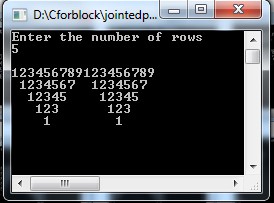
The C program allows the user to enter the number of rows then it displays two combined upside-down pyramid number pattern according to the number of rows.
Similar post
Java program to the combined Pyramid pattern using while loop
C++ program to the combined Pyramid pattern using while loop
Suggested for you
Input and output function in C language