Nested if statement in C programming language
Nested if statement in C programming language
We will learn about Nested if statement in C programming language
In C Programming Language, the nested if-else statement is one if-else statement is used inside another if- else statement.
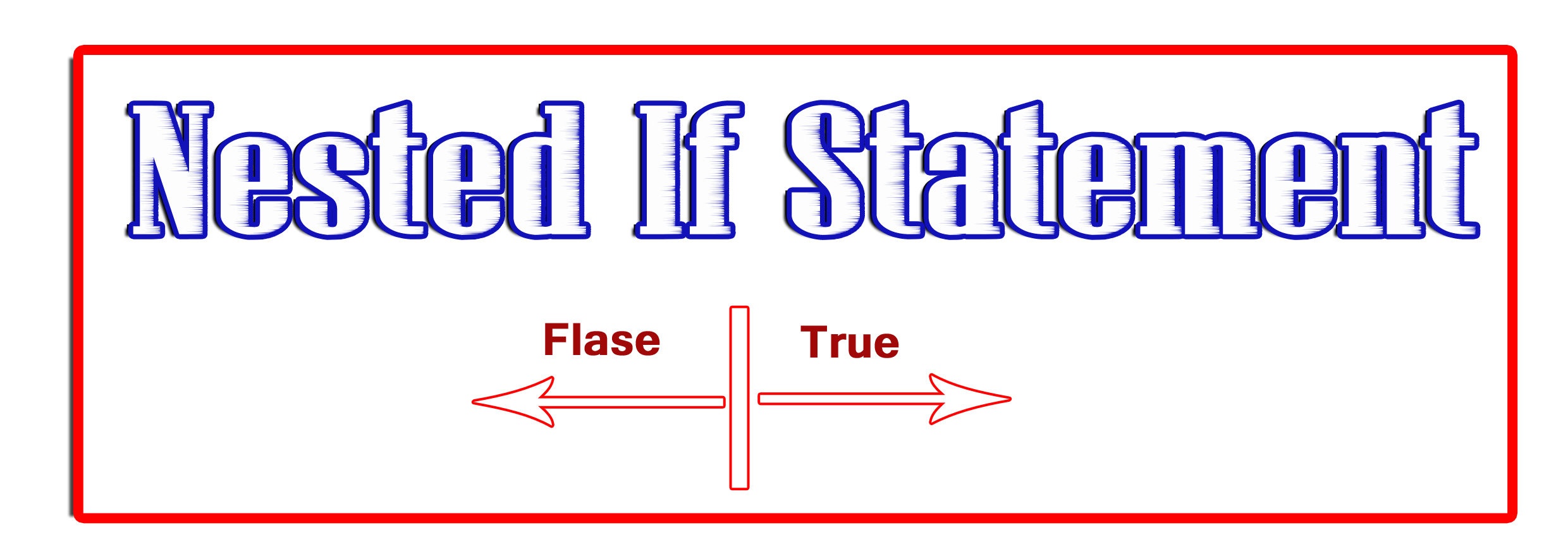
Declaration
The syntax of Nested if in C Language
if(condition1) //outer if {//executes when condition1 is true if(condition1)// inner if { //executes when condition2 is true } }
Nested if statement in C
Explanation of nested if with else statements
if(Test_expression1) { //outer if statement(s); //when the test_expression1 is true //executes this statements if(Test_expression2) { //inner if statement(s); //when the test_expression2 is true //executes this statements } else{ statement(s); //when the test_expression2 is false //executes this statements } } else{ statement(s); //when the test_expression1 is false //executes this statements }
Explain the nested if – else loop

Flow diagram of nested if else in C Language
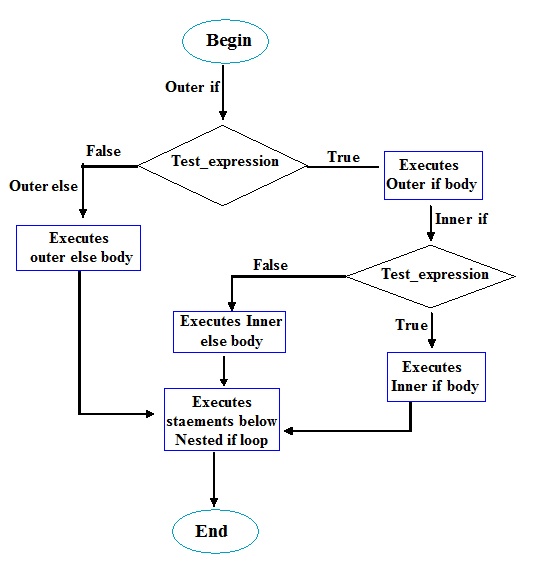
How works nested if statements
In nested if statements, initially, the test expression of outer if the loop is evaluated.When the condition of outer if becomes true, the if part statement is executed and the output displayed. Then, the flow of control jumps to the inner– if loop and evaluates test expression of inner if
the test expression of inner if the loop is evaluated. when the condition of inner if becomes true, the statements of if is executed. When the condition is false, else part is executed then else statement is displayed. Then nested if loop may be an exit or terminated.
Program 1
#include <stdio.h> #include <stdlib.h> int main() { int age; printf("Enter your age Here\n"); scanf("%d",&age); //get input if(age<13){ // if statement printf("you are a child!\n"); } else if(age<35){ //elseif statement printf("you are a younger!\n"); } else{ //else statement printf("you are a older!\n"); } getch(); return 0; }
When the above code is compiled and executed, it produces the following results
Enter your age 23 you are a younger
Program 2
#include <stdio.h> #include <stdlib.h> int main() { int a,b,c; printf("Enter the first number for a..:\n"); scanf("%d",&a); printf("Enter the second number for b..:\n"); scanf("%d",&b); printf("Enter the third number for c..:\n"); scanf("%d",&c); if(a>b){ if(a>c){ printf("%d is a large number\n",a); } else{ printf("%d is less than number %d and %d\n",a,c); } } else{ printf("%d is a less than number %d\n",a,b); } getch(); return 0; }
When the above code is compiled and executed, it produces the following results
Enter the first number a.. 1 Enter the second number b.. 2 Enter the third number c.. 3 1 is less than 2
Program 3
#include <stdio.h> #include <stdlib.h> int main() { int age; float Zscore; printf("Enter your age\n"); scanf("%d",&age); printf("Enter your Zscore\n"); scanf("%f",&Zscore); if(age>18){//outer if printf("eligible for university\n"); if(Zscore>1.0){ //inner if printf("qualified for university\n"); } else{ //inner else printf("Not qualified for university\n"); } } else{ // outer else printf("Not eligible for university\n"); } getch(); return 0; }
When the above code is compiled and executed, it produces the following results
Enter your age 19 Enter your Zscore 1.01 eligible for university qualified for university
Suggested for you
If statement in Python language
Electricity bill calculation program Java language
Electricity bill calculation program Python language
Electricity bill calculation program C language
Electricity bill calculation program C++ language
Electricity bill calculation program using Java method
Electricity bill calculation program using C function