Java program to display all odd or even numbers 1 to n with label
- Home
- Find elements
- Java program to display all odd or even numbers 1 to n with label
- On
- By
- 0 Comment
- Categories: Find elements
Java program to display all odd or even numbers 1 to n with label
Java program to display all odd or even numbers 1 to n with label
In this article, we will discuss the concept of Java program to display all odd or even numbers 1 to n with label using loops
In this post, we will learn how to find and display odd and even numbers with label using the loop.
Here, we will use for loop, while loop, and do-while loop to find and display odd and even numbers from 1 to n in Java programming language.
Program 1
Java program to find odd and even numbers from 1 to n
Using the for loop
This program allows the user to enter the maximum numbers. It finds and displays even and odd numbers with the label from 1 to entered number using for loop
import java.util.Scanner; class OddEvenWithLabel{ public static void main(String args[]){ Scanner scan=new Scanner(System.in); //create a scanner object for input System.out.print("Enter the random number: "); int num=scan.nextInt();//get input from the user for num1 System.out.print("Display all odd and even number till "+num); System.out.print("\n\n"); for(int i=1; i<=num; i++){ if(i%2==0){ System.out.print(i+" Is Even"); System.out.print("\t"); } else{ System.out.print(i+" Is Odd\t"); } } } }
When the above code is executed, it produces the following results
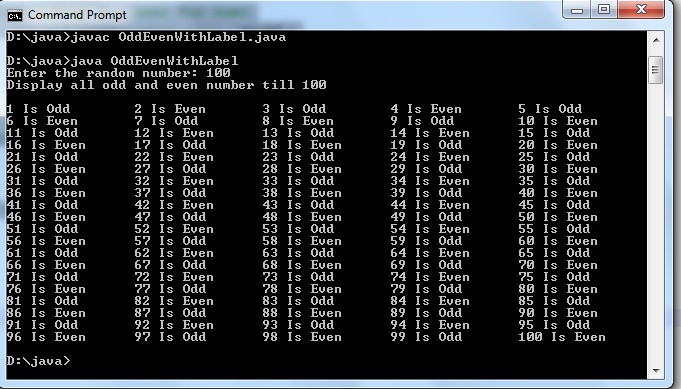
Program 2
This program allows the user to enter the maximum numbers. It finds and displays even and odd numbers with the label from 1 to entered number using while loop.
Using the while loop
import java.util.Scanner; class OddEvenWithLabel1{ public static void main(String args[]){ Scanner scan=new Scanner(System.in); //create a scanner object for input System.out.print("Enter the random number: "); int num=scan.nextInt();//get input from the user for num1 System.out.print("Display all odd and even number till "+num); System.out.print("\n\n"); int i=1; while( i<=num){ if(i%2==0){ System.out.print(i+" Is Even"); System.out.print("\t"); } else{ System.out.print(i+" Is Odd\t"); } i++; } } }
When the above code is executed, it produces the following results
Using the do-while loop
Program 3
This program allows the user to enter the maximum numbers. It finds and displays even and odd numbers with label 1 to entered number using the do-while loop.
import java.util.Scanner; class OddEvenWithLabel2{ public static void main(String args[]){ Scanner scan=new Scanner(System.in); //create a scanner object for input System.out.print("Enter the random number: "); int num=scan.nextInt();//get input from the user for num1 System.out.print("Display all odd and even number till "+num); System.out.print("\n\n"); int i=1; do{ if(i%2==0){ System.out.print(i+" Is Even"); System.out.print("\t"); } else{ System.out.print(i+" Is Odd\t"); }i++; }while( i<=num); } }
When the above code is executed, it produces the following results
Similar post
C program to find the number is odd or even with label using loops
C++ program to find the number is odd or even with label using loops
Python program to find the number is odd or even with label using loops
Java program to find the number is odd or even with label using loops
C++ program to find odd or even number using switch statements
C program to find odd or even number using switch statements
Java program to check odd and even using recursion
C program to check odd and even using recursion
C++ program to check odd and even using recursion
Python program to check odd and even using recursion
Python program to check odd and even using function
Java program to check odd and even using Method
C program to check odd and even using function
Suggested for you