Java code to Floyd’s triangle star pattern
- Home
- Floyd's triangle
- Java code to Floyd’s triangle star pattern
- On
- By
- 0 Comment
- Categories: Floyd's triangle, star pattern
Java code to Floyd’s triangle star pattern
Java code to triangle star pattern
In this tutorial, we will discuss Java code to Floyd’s triangle star pattern using nested for loop.
We will learn how to create Floyd’s triangle pattern in Java programming language using for loop
Pattern 1
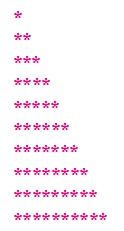
Java Code for Floyd’s triangle program 1
Java program to display the right triangle star pattern
import java.util.Scanner; public class JavaStarPattern{ public static void main(String args[]){ Scanner sc=new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows=sc.nextInt();//get input from user for(int i=0; i<=rows; i++){ for(int j=0; j<=i; j++){ System.out.print("*");//print star } System.out.println(); } } }
When the above code is executed, it produces the following results:
Enter the number of rows: 9 * ** *** **** ***** ****** ******* ******** ********* **********
Pattern 2
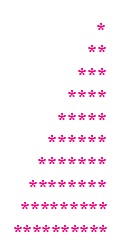
Java Code for Floyd’s triangle program 2
Java program to display mirrored right triangle star pattern
import java.util.Scanner; public class JavaStarPattern{ public static void main(String args[]){ Scanner sc=new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows=sc.nextInt(); for(int i=1; i<=rows; i++){//parent for loop for print rows for(int j=1; j<=rows-i; j++){ System.out.print(" ");//print space } for(int k=1; k<=i; k++){ System.out.print("*");//print star } System.out.println();//move to next line } } }
When the above code is executed, it produces the following results:
Enter the number of rows: 9 * ** *** **** ***** ****** ******* ******** *********
Pattern 3
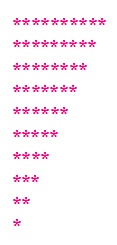
Java Code for Floyd’s triangle program 3
Java program to display reverse right triangle star pattern
import java.util.Scanner; public class JavaStarPattern{ public static void main(String args[]){ Scanner sc=new Scanner(System.in); System.out.print("Enter the number of rows\n: "); int rows=sc.nextInt();//get input from user for(int i=rows; i>=1; i--){ for(int j=1; j<=i; j++){ System.out.print("*"); /print star } System.out.println(); } } }
When the above code is executed, it produces the following results:
Enter the number of rows: 9 ********* ******** ******* ****** ***** **** *** ** *
Pattern 4
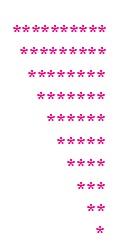
Code for Floyd’s triangle program 4
Program to display reverse mirrored right triangle star pattern
import java.util.Scanner; public class JavaStarPattern{ public static void main(String args[]){ Scanner sc=new Scanner(System.in); System.out.print("Enter the number of rows: "); int rows=sc.nextInt(); for(int i=1; i<=rows; i++){//parent for loop lterate rows for(int j=1; j<=i; j++){ System.out.print(" ");//print space } for(int k=i; k<=rows; k++){ System.out.print("*");//print star } System.out.println();//move to next line } } }
When the above code is executed, it produces the following results:
Enter te number of rows: 9 ********* ******** ******* ****** ***** **** *** ** *
Similar post
Floyd’s triangle number pattern using for loop in C
Floyd’s triangle pattern using nested for loop in Java
Floyd’s triangle pattern using nested while loop in Java
Hollow pyramid triangle pattern in C++ language
Suggested for you
Nested for loop in Java language
Nested for loop in C++ language
Nested for loop in Python language