If statement in C programming language
If statements in C programming language
In this tutorial, we will discuss If statement in C programming language
if …else…statement
Three type of “if“ statements are mainly available in Java:
- if statements
- if else statements
- if else if else statements
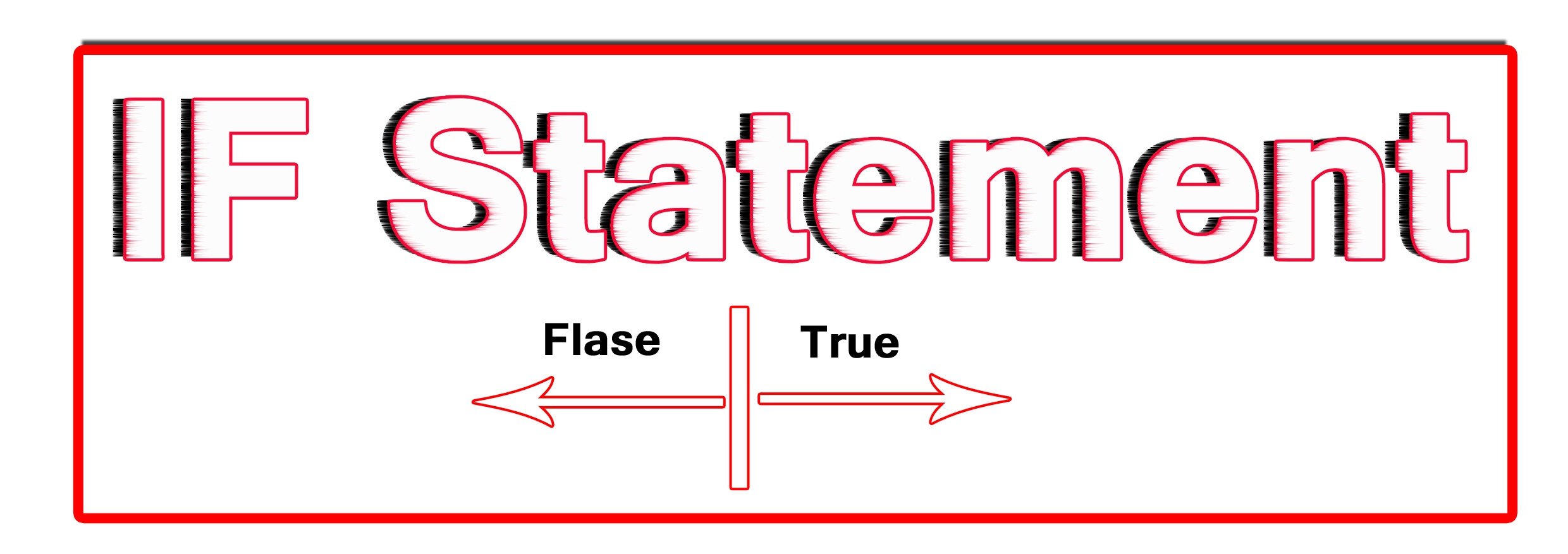
if statements
Declaration
Syntax
if(Test_condition){ statement(s) } //this statements are false control flow jump out of if part
}
Flow diagram – if in C language
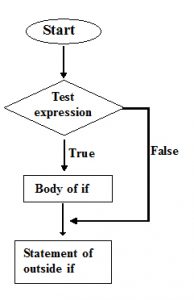
In the C programming language, first test the Boolean expression inside the parenthesis of if part, when the Boolean expression is true, flow of control enters the codes inside the body of if part and executes body part statement, when the Boolean expression is false, flow loop control skips the body of if part and comes out loop control.
Example
How if statement works in C
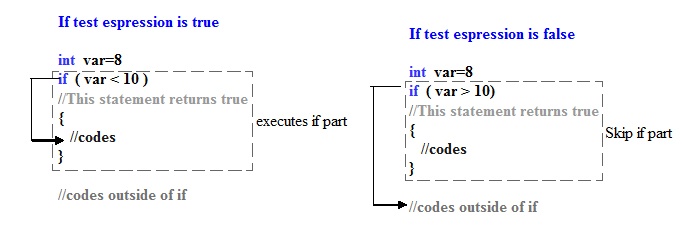
Program 1
#include <stdio.h> #include <stdlib.h> int main() { int marks=66; if(marks>=65) { printf("Happy you pass exam\n"); } printf("End of loop\n"); getch(); return 0; }
In the above program, initially, marks are declared 66.
the test expression is marks>=65
When the test expression is evaluated, test expression becomes true. So the body of if part statement is executed and display “Happy you pass exam” .
Then the loop control exit from if part and executes out of if statements
When the above code is compiled and executed, it produces the following results
Happy you pass exam End of loop
In the above program, initially marks is declared 66. when the mark is 66, the test expression (marks>=65) is evaluated and it returns true, the code inside the body of if statements executed.
Now, when we change the value of marks to below 65, the output of in this case will be executed
End of loop
In the example, when the mark is 60, the test expression (marks>=65) is evaluated and returns false hence the flow of control skips the execution of the body of if statements and flow of control comes out of if body for execution
In the above program statements of the end of the loop is always executed.
if else in C language
Declaration
Syntax
if(Test_condition){ statement(s) } else{ statement(s)
}
Flow diagram – if else in C language
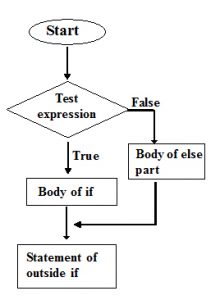
In the C programming language, first evaluates the test expression inside the parenthesis of if part, when the test expression is true, the flow of control enters the codes inside the body of if part and executes body part statement. When the test expression is false, loop control skips the body of if part and goes to else part and execute its statements.
How if -else statements works in C
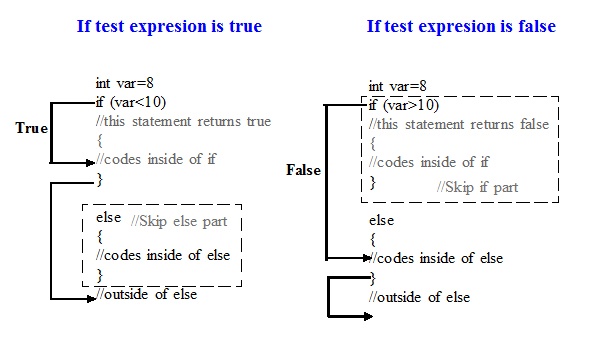
Program 1
#include <stdio.h> #include <stdlib.h> int main() { int age; printf("Enter your age..."); scanf("%d",&age); if(age<=18) { printf("your age is %d you are a younger\n",age); } else{ printf("your age is overage",age); } getch(); return 0; }
When the above code is compiled and executed, it produces the following results.
Enter your age .... 13 your age is 14 you area younger
if …else if.. else…statement
Declaration
Syntax
if(Test_condition){ statement(s) } else if{ statement(s)
}
else{ statement(s)
}
In the C programming language, first evaluates the test expression inside the parenthesis of if part, when the test expression is true, the flow of control enters the body of if part and executes body part statement. When the test expression is false, loop control skips the codes inside the body of if part and goes to else if the part to execute its statements.
When the test expression is evaluated(else if) to be false, else part executes and exits from the loop for rest.
Flow chart of if else if else in C language
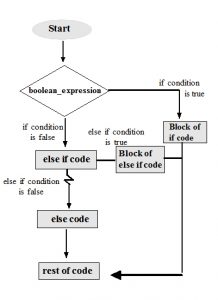
How if -else statements works in C
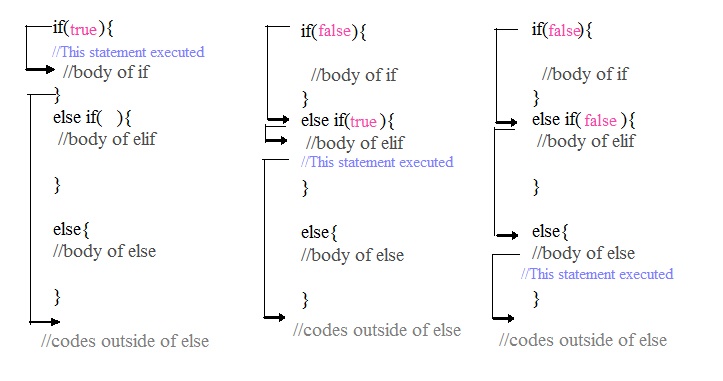
Program 1
#include <stdio.h> #include <stdlib.h> int main() { int age; printf("Enter your age here"); scanf("%d",&age); if(age<=13) { printf("your age a chuld\n"); } else if(age<35){ printf("your age is younger"); } else{ printf("your age is older"); } getch(); return 0; }
When the above code compiled and executed, it produced the following results
Enter your age Here
45
you are an older
Similar post
If statement in Python language
Suggested for you
Nested for loop in C++ language
Nested while loop in C++ language
Nested for loop in Java language
Nested while loop in Java language
Three dim Array in C++ language
Single dim Array in Java language
Two dim Array in Java language
Three dim Array in Java language
Single dim Array in C language