Function to subtract two numbers in PHP
- Home
- Calculations
- Function to subtract two numbers in PHP
- On
- By
- 0 Comment
- Categories: Calculations, subtraction
Function to subtract two numbers in PHP
Function to subtract two numbers in PHP
In this tutorial, we will discuss the concept of Function to subtract two numbers in PHP
In this topic, we are going to learn how to write a program to subtract two numbers using user-defined function in PHP programming language
Find Difference of two numbers
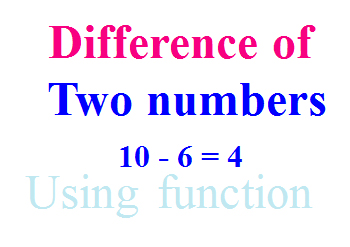
Find difference of two numbers using function – #1
In this program, the user declare two integer variables $x and $y as parameters of the user defined function. Then two numbers are subtracted first value from second value using minus(-) operator. finally when the user call the function with argument, the result is displayed on the screen
Program 1
<?php //php script //function definition function subTwoNumbers($x, $y) { return $x - $y;//calculate the difference using minus } //Calling the function and print result echo "The difference of 200 and 30: ".subTwoNumbers(200,30); echo "<br>"; echo "The difference of 20 and 30: ".subTwoNumbers(20,30); echo "<br>"; echo "The difference of 10 and 110: ".subTwoNumbers(10,110); echo "<br>"; echo "The difference of -100 and -80: ".subTwoNumbers(-100,-80); echo "<br>"; //exit php ?>
When the above code is executed, it produces the following result
The difference of 200 and 30: 170 The difference of 20 and 30: -10 The difference of 10 and 110: -100 The difference of -100 and -80: -20
Find difference of two numbers using function – #2
In this program, the user declare two integer variables $x and $y as parameters of the function. Then two numbers are subtracted first value from second value using minus(-) operator. finally when the user call the function with argument, the result is displayed on the screen
Program 2
<?php //php script //function definition function subTwoNumbers($x, $y) { $sub=$x - $y; //calculate difference using minus operator return $sub;//return the result echo $sub;//print the result when calling the function } //Calling the function and print result echo "The Difference of 20 and 300: "; echo subTwoNumbers(20,300); echo "<br>"; echo "The Difference of 200 and 120: "; echo subTwoNumbers(200,120); echo "<br>"; echo "The Difference of 10 and -190: "; echo subTwoNumbers(10,-190); echo "<br>"; //exit php ?>
When the above code is executed, it produces the following result
The Difference of 20 and 300: -280 The Difference of 200 and 120: 80 The Difference of 10 and -190: 200
Suggested for you
Subtraction of two numbers in Java language
Subtraction of two numbers in C Language
Subtraction of two numbers in C++ Language
Subtraction of two numbers in Python Language
Subtraction of two numbers using method in Java language
Subtraction of two numbers using function in C Language
Subtraction of two numbers using function in C++ Language
Subtraction of two numbers using function in Python Language
PHP Addition of two numbers using function