function in C programming language with example
- Home
- function in C
- function in C programming language with example
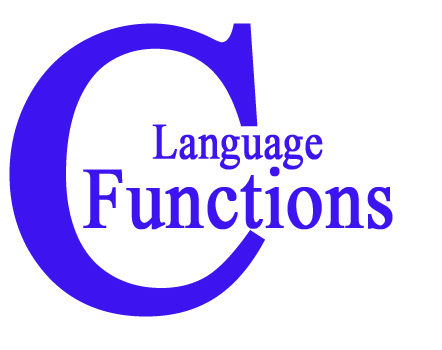
- On
- By
- 0 Comment
- Categories: function in C
function in C programming language with example
Functions in C programming language with example
In the tutorial, we will discuss function in C programming language with examples. The function is the building block of programming in C language.
Description
Description of function in C programming language
What is a function?
The function is a block of statement or instruction enclosed by curly braces { } that together perform one of the special tasks in C program. Collection of these functions perform a special purpose(inter-connected a lot of tasks) in C program.
return_type function_name (parameter list){
//function body
return (expression)
}
Explanation of function
return_type – The return type is a data type used for the function declaration.
Ex – int, float, double, void
Void data type never returns any value but the usage of other data type returns the value.
function_name – function name is used to identify the function in the C Programming language. Every function should have a name(user define function) as the function name represents the uniqueness of function and function name is used to call the function.
parameter_list – A function may contain a parameter list(one or more parameter). Sometimes, functions may not contain a parameter(it is optional). However, when a function is executed, you pass a value(it’s called argument) to the parameter list which refers to the parameter type, order of parameter and number of the parameter of a function.
Function_body – The body of the function consists of a group of statements. They will be invoked when the function is called.
Type of functions in C language
Two types of function available in C language.
Standard library function – standard library functions are built-in functions or predefined functions in C language to handle a special task.
Ex – mathematical operations, I/O processing and string handling etc
These functions are already defined in the header file of C programming language. When they are included in the header file of the program, we can use these function easily.
Ex –
string.h – string handling functions
math.h – Arithmetic operations
The printf() function is a predefined function in C language used to get the output on the screen. This function is defined in “stdio.h” header file.
The scanf() function is a predefined function in C language used to get the input from the user. This function is defined in “stdio.h” header file.
User defined function in C language
C language supports you to define functions according to your needs and satisfaction. These functions are called as user-defined functions in C language
4 type of function available in C Language
- Function with no argument and no return value
- Function with no argument and with return value
- Function with argument and no return value
- Function with argument and return value
Examples
- void add() //No return without argument
- void sub(int,int)//No return argument
- int mul() //Return without argument
- float div(int ,int) //Return with argument
Add two numbers using function(No return without argument)
#include <stdio.h> #include <stdlib.h> void add(); //function declaration void main() { add(); //calling function no argument getch(); return 0; } void add()//no argument is passed to this function {//void data type never return anything from function int a,b; printf("Enter first number for a"); scanf("%d",&a); printf("Enter second number for b"); scanf("%d",&b); int c=a+b; printf("total value (a+b) is %d\n",c); }
When the above program is compiled and run, it will produce the following result
Enter first number for a :45 Enter second number for b :78 Total value (a+b) is 123
Calculating the difference between two numbers using function(No return with argument)
#include <stdio.h> #include <stdlib.h> void sub(int f_num, int s_num);//function declaration int main() { sub(67,34); getch(); return 0; } void sub(int f_num, int s_num) //No argument is passed to this function { //void data type never return value from function printf("Enter the first number of a: "); scanf("%d",&f_num); printf("Enter the first number of b: "); scanf("%d",&s_num); int diff=f_num-s_num; printf("Total value(a+b) is %d\n",diff); }
When the above program is compiled and run, it will produce the following result
Enter your first number :67 Enter your second number :34 Different between two numbers :33
Multiplication of two numbers using function(Return without argument)
#include <stdio.h> #include <stdlib.h> int mul();//function declaration int main() { printf("Answer is: %d",mul()); getch(); return 0; } int mul() //No argument is passed to this function { //void data type never return value from function int f_no, s_no; printf("Enter the first number of f_no: "); scanf("%d",&f_no); printf("Enter the first number of s_no: "); scanf("%d",&s_no); return f_no*s_no; }
When the above program is compiled and run, it will produce the following result
Enter first number for f_no:12 Enter second number for s_no:23 Answer is :276
Multiplication of two numbers using function(Return with arguments
#include <stdio.h> #include <stdlib.h> int mul(int a, int b);//function declaration int main() { printf("The result is: %d",mul(45,34));//function calling getch(); return 0; } int mul(int f_no, int s_no) //No argument is passed to this function { //void data type never return value from function printf("Enter the first number of f_no: "); scanf("%d",&f_no); printf("Enter the first number of s_no: "); scanf("%d",&s_no); return f_no*s_no;//return statements in function }
When the above program is compiled and run, it will produce the following result
The first number of f_no: 45 The second number of s_no: 100 The result is :4500
Suggested for you
suggested for you
User defined function in C language
Arithmetic functions in C Language