Find the product of two numbers using recursion in C++
- Home
- Find elements
- Find the product of two numbers using recursion in C++
- On
- By
- 0 Comment
- Categories: Find elements
Find the product of two numbers using recursion in C++
Find the product of two numbers using recursion in C++
In this tutorial, we will discuss a concept of Find product of two numbers in C++ using recursion
In this article, we are going to learn how to Find product of two numbers using recursion in the C++ programming language
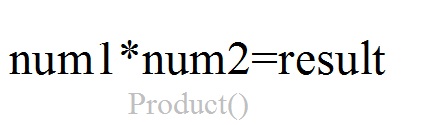
program
This program allows the entry of two digits from the user and to find the product of two numbers using the recursive function in C++ programming language.
#include <iostream> #include <conio.h> using namespace std; int product(int,int); //function prototype / declaration int main() { int num1,num2,result; cout<<"Enter two number to find their product\n"; cin>>num1>>num2; //numbers receive from the user result=product(num1,num2);//assign the output to variable result //function call cout<<"Product of "<<num1<<" and "<<num2<<" is "<<result; getch(); return 0; } int product(int a, int b) { if(a<b) { return product(b,a); } else if(b!=0){ return (a+product(a,b-1)); } else{ return 0; } }
When the above code is executed, it produces the following results
Enter two numbers to find their product 30 12 Product of 30 and 12 is: 360
Method
- Declare three variables num1, num2 and result as “int” type.
- Receive two numbers from user and store variable as num1 and num2 respectively.
- Call the function and assign the output value to variable result.
- Product() function is used to calculate the product of of two numbers.
- Display the result on the screen.
Similar post
Calculate the product of two numbers in C using recursion
Calculate the product of two numbers in Java using recursion
Calculate the product of two numbers in Python using recursion
Suggested for you
No Comments
Multiply The Odd Numbers From A File Using A Recursive Function – ContractWebsites.com SubReddits February 14, 2021 at 7:44 am
[…] numbers from the file are: 7 3 4 2 1 8 6 5 0 9 I tried some methods from this site https://code4coding….recursion-in-c/ but it refers to just 2 numbers while I have more than 2 in my file I would appreciate if you could […]