Example to subtract two integer using pointer in C
- Home
- Calculations
- Example to subtract two integer using pointer in C
- On
- By
- 0 Comment
- Categories: Calculations, subtraction
Example to subtract two integer using pointer in C
Example to subtract two integer using pointer in C
In this article, we will discuss the concept of the Example to subtract two integer using pointer in C programming language
In this post, we are going to learn how to write a program to find the subtraction of two numbers using pointer in C programming language
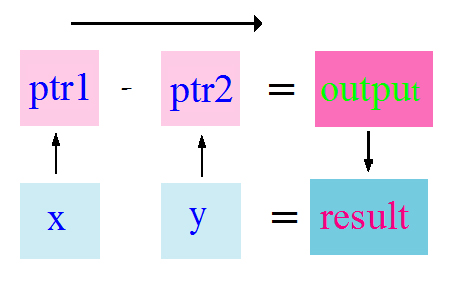
Code to find the subtraction of two numbers
Subtract two integer using pointer
The program use to calculate subtraction of given two integer numbers using pointer in C language
Program 1
#include <stdio.h> #include <stdlib.h> int main() { int num1,num2; //declare variables num1,num2 int *ptr1,*ptr2; //declare pointer variable int sub; num1=350; //variable initialization num2=50; ptr1=&num1; //assign the address of the variable to pointer variable ptr2=&num2; sub=*ptr1 - *ptr2; //calculate the subtraction using pointer printf("Subtraction of the given two integer values is: %d",sub); //display result on the screen getch(); return 0; }
When the above code is executed, it produces the following result
Subtraction of the given two integer values is: 300
Subtract two integer using pointer – takes input from the user
The program allow the user to enter two numbers and then calculates subtraction of given two integer numbers using pointer in C language
Program 2
#include <stdio.h> #include <stdlib.h> int main() { int num1,num2; //1 int *ptr1,*ptr2; //2 int sub; //3 printf("Please enter value for num1: "); //4 scanf("%d",&num1); //5 printf("Please enter value for num2: "); //6 scanf("%d",&num2); //7 ptr1=&num1; //8 ptr2=&num2; sub=*ptr1 - *ptr2; //10 printf("Subtraction of the two integer values is: %d",mul); //11 getch(); return 0; }
When the above code is executed, it produces the following result
Please enter value for num1: 1000 Please enter value for num1: 700 Subtraction of the two integer values is: 300
Methods
- Create two variables to store two numbers as input provided by the user: num1,num2;
- Create two pointer variables (*ptr1,*ptr2)to store the address of the numbers: num 1 and num2
- Create a variable to store the subtraction of these numbers: sub
- Request the user to enter first input for store variable num1
- Using scanf() function store the first input value in num1
- Request the user to enter second input for store variable num2
- Using scanf() function store the second input value in num2
- Assign the address of variable num1 to ptr1
- Assign the address of variable num2 to ptr2
- Find the subtraction of two number using their addresses – find the subtraction of num1,num2 using their addresses and add them using the pointer variables
- Finally, display result on the screen- the sum of both numbers num1 and num2
Similar post
C program find the sum of two numbers using the function
C program find the sum of two numbers using recursion
C program to find the product of two numbers using the pointer
Suggested for you
Input-output function in C language