Example for Python program to check Prime number
- Home
- Check value
- Example for Python program to check Prime number
- On
- By
- 0 Comment
- Categories: Check value
Example for Python program to check Prime number
Example for Python program to check Prime number
In this tutorial, we will discuss the concept of the Example to Python program to check the Prime number
In this post, we are going to learn how to check a number is prime or not using for and while loop in Python programming language :
Prime number
The number that can be divided by 1 and itself. it is called prime number
for Example 2,3,5,7,11,13….
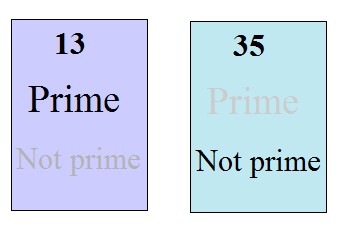
To understand this example programs, you should have previous knowledge of following Python topics
If statements in Python language
Example Python program
Find prime number using for loop
Program 1
This program allows the user to enter a positive number and then it will check the given number is a prime number or not using for loop in Python language
#Python program to check Prime number #Takes input from user num=int(input("Enter a number: ")) if num>1: for i in range(2,num): if (num % i)==0: print(num, " is not prime number") break; else: print(num, " is a prime number") #if the input number is lessthan or equal to 1 else: print(num, " is not prime number")
When the above code is executed, it produces the following results
Case 1
Enter a number: 11 11 is a prime number
Case 2
Enter a number: 12 12 is not prime number
Case 3
Enter a number: 1 1 is not prime number
Case 4
Enter a number: -10 10 is not prime number
Find prime number using while loop
Program 2
This program allows the user to enter a positive number and then it will check the given number is a prime number or not using while loop in Python language
#Python program to check Prime number #Takes input from user num=int(input("Enter a number")) count=0 i=2 while(i<=num/2): if (num % i)==0: count=count+1 break; i=i+1 if(count==0 and num!=1): print(" %d is a prime number"%num) else: print(" %d is not aprime number"%num)
When the above code is executed, it produces the following results
Case 1
Enter a number13 13 is a prime number
Case 2
Enter a number15 15 is not a prime number
Suggested for you
Similar post
Program to check whether a Number is Prime or Not in C++
Program to check whether a Number is Prime or Not in C
Program to check whether a Number is Prime or Not in Java
Program to check whether a Number is Prime or Not in Python