Example program to check whether a Number is Prime or Not in Java
- Home
- Check value
- Example program to check whether a Number is Prime or Not in Java
- On
- By
- 0 Comment
- Categories: Check value
Example program to check whether a Number is Prime or Not in Java
Example program to check whether a Number is Prime or Not in Java
In this tutorial, we will discuss the concept of an Example program to check whether a Number is Prime or Not in Java
In this post, we are going to learn how to check a number is prime or not using for, while, and do-while loop in Java programming language:
The number that can be divided by 1 and itself. it is called prime number
for Example 2,3,5,7,11,13….
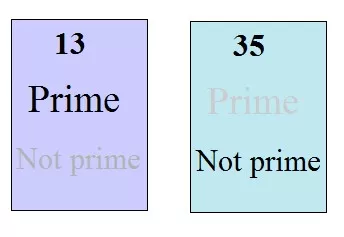
To understand this example program, you should have previous knowledge of the following Java topics
do-while loop in Java language
Example program to check whether a Number is Prime or Not
Find the prime number using the for loop
In this program, we will check whether the given number is prime or not, using for loop, first, the user is asked to enter a number randomly, then the program checks if the given number is prime or not
Program 1
import java.util.Scanner; class Check_Prime{ public static void main(String args[]){ int num; boolean isPrime=false; Scanner scan=new Scanner(System.in); //create a scanner object for input System.out.print("Enter a number: "); num=scan.nextInt();//get input from the user for num1 for(int i=2; i<=num/2; i++){ //condition for non-prime if(num%i==0) { isPrime=true; break; } } if(!isPrime){ System.out.println(num+" is a prime numbner "); } else{ System.out.println(num+" is not a prime numbner "); } } }
When the above code is executed, it produces the following results
Case 1
Enter a number: 23 23 is a prime number
Case 2
Enter a number: 54 54 is not a prime number
This program allows the user to enter a positive number and then it will check the given number is a prime number or not using for loop in Java language
Find the prime number using the while loop
In this program, we will check whether the given number is prime or not, using while loop, first, the user is asked to enter a number randomly, then the program checks if the given number is prime or not
Program 2
import java.util.Scanner; class Check_Prime1{ public static void main(String args[]){ int num; boolean isPrime=false; Scanner scan=new Scanner(System.in); //create a scanner object for input System.out.print("Enter a number: "); num=scan.nextInt();//get input from the user for num1 int i=2; while( i<=num/2){ //condition for non-prime if(num%i==0) { isPrime=true; break; } i++; } if(!isPrime){ System.out.println(num+" is a prime numbner "); } else{ System.out.println(num+" is not a prime numbner "); } } }
When the above code is executed, it produces the following results
Case 1
Enter a number: 12 12 is not a prime number
Case 2
Enter a number: 37 37 is a prime number
This program allows the user to enter a positive number and then it will check whether the given number is a prime number or not, using a while loop in the Java language
Find the prime number using the do-while loop
In this program, we will check whether the given number is prime or not, using do-while loop, first, the user is asked to enter a number randomly, then the program checks if the given number is prime or not
Program 3
import java.util.Scanner; class Check_Prime2{ public static void main(String args[]){ int num; boolean isPrime=false; Scanner scan=new Scanner(System.in); //create a scanner object for input System.out.print("Enter a number: "); num=scan.nextInt();//get input from the user for num1 int i=2; do{ //condition for non-prime if(num%i==0) { isPrime=true; break; } i++; }while( i<=num/2); if(!isPrime){ System.out.println(num+" is a prime numbner "); } else{ System.out.println(num+" is not a prime numbner "); } } }
When the above code is executed, it produces the following results
Case 1
Enter a number: 100 100 is not a prime number
Case 2
Enter a number: 101 101 is not a prime number
This program allows the user to enter a positive number and then it will check whether the given number is a prime number or not using the do-while loop in the Java language
Suggested for you
Data type and variable in Java language
Similar post
Program to check whether a Number is Prime or Not in C++
Program to check whether a Number is Prime or Not in C