Electricity bill Calculation program using function in Cpp
- Home
- Calculations
- Electricity bill Calculation program using function in Cpp
- On
- By
- 0 Comment
- Categories: Calculations
Electricity bill Calculation program using function in Cpp
Electricity bill calculation program using the function in Cpp
In this tutorial, we will discuss the Cpp program to Electricity bill calculation using the function
In this article, we will discuss how to calculate electricity bill in Cpp programming language using the function
We can calculate monthly electric power usage in many ways in the C++ programming language.
In this tutorial, we will write two programs calculating electricity bill in C++ language.
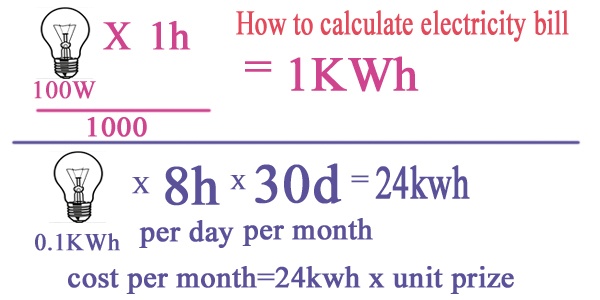
Before we write a program to calculate the electricity bill, we must understand electricity charges and rates.
/*1 - 100 unit - 1.5/= 101-200 unit - 2/= 201-300 unit - 3/= above 300 - 4/=*/
In my previous post, I have explained to the C++ program to calculate the electricity bill
However, in this program, we embed the logic of the Calculate electricity bill program in the function
Once this program received the units and it will calculate the electricity unit and cost per month
After receiving the input from the user, it is stored in the variable of the unit and then program calculate electricity bill
This program can have the following steps for completion of this program
- Declare total unit consumed by the customer using the variable unit.
- If the unit consumed less or equal to 50 units, calculates the total amount of usage =unit*1.50
- If unit consumed between 50 to 150 units, calculates the total amount of usage =(50*1.5)+(unit-50)*2)
- If unit consumed between 150 to 250 units ,calculates total amount of usage =(50*1.5)+(100*2)+(unit-150)*3
- If unit consumed above 250 units ,calculates total amount of usage =(50*1.5)+(100*2)+(100)*3+(unit-250)*4
- No additional charge
Without && operator
Program 1
#include <iostream> #include <conio.h> using namespace std; int calc_Electricity(int);//function prototype int main() { int unit=0; cout<<"Enter total units consumed: "; cin>>unit;//Reads input from user and stored in variable unit calc_Electricity(unit);//function call getch(); return 0; } int calc_Electricity(int unit){//function definition double amount; if(unit<=50) {//below 50 units amount=unit*1.50; } else if(unit<=150) {//below 150 units amount=((50*1.5)+(unit-50)*2.00); } else if((unit<=250)){//below 250 units amount=(50*1.5)+((150-50)*2.00)+(unit-150)*3.00; } else{//above 250 units amount=(50*1.5)+((150-50)*2.00)+((250-150)*3.00)+(unit-250)*4; } cout<<"Electricity bill = Rs."<<amount; //display the amount of electricity bill per month on the screen }
When the above code is executed, it produces the following results
Enter total units consumed: 300 Electricity bill = Rs.775
With && operator
Program 2
#include <iostream> #include <conio.h> using namespace std; int calc_Electricity(int);//function prototype int main() { //rates apply // 1- 50 units - 1.50 //51- 150 units - 2.00 //101 - 250 units - 3.00 //above 251 units - 4.00 int unit=0; cout<<"Enter total units consumed\n"; cin>>unit; //Reads input from user and store in variable unit calc_Electricity(unit);//function call getch(); return 0; } int calc_Electricity(int unit){//function definition double amount; if((unit>=1)&&(unit<=50))//between 1 - 50 units { amount=unit*1.50; } else if((unit>50)&&(unit<=150))//between 50 150 units { amount=((50*1.5)+(unit-50)*2.00); } else if((unit>150)&&(unit<=250)){//between 150 - 250 units amount=(50*1.5)+((150-50)*2.00)+(unit-150)*3.00; } else if(unit>250){//above 250 units amount=(50*1.5)+((150-50)*2.00)+((250-150)*3.00)+(unit-250)*4; } else{ cout<<"No usage "; amount=0; } cout<<"Electricity bill = Rs"<<amount; }
When the above code is executed, it produces the following results
Similar post
C++ program to calculate electricity bill
C program to calculate electricity bill
Java program to calculate electricity bill
Python program to calculate electricity bill
C++ program to calculate electricity bill using function
C program to calculate electricity bill using function
Java program to calculate electricity bill using method
Python program to calculate electricity bill using function
Suggested for you
Type of User-define function in C++ language