Cpp Program to display smallest among three numbers
- Home
- Find elements
- Cpp Program to display smallest among three numbers
- On
- By
- 0 Comment
- Categories: Find elements
Cpp Program to display smallest among three numbers
Cpp Program to display smallest among three numbers
In this tutorial, we will discuss the Cpp Program to display the smallest among the three numbers.
In this program, we will discuss a simple concept of the program to find the smallest number among three numbers in the Cpp programming language.
In this topic, we learn how to find the smallest number from given three numbers using if statements.
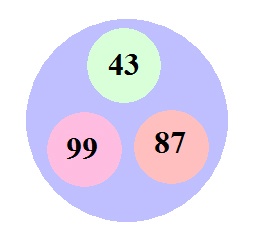
Find the smallest number using if statements
Among integer numbers
Program 1
#include <iostream> #include <conio.h> using namespace std; int main() { int num1,num2,num3; //declare the variables cout << "Enter the first number: "; cin>>num1;//get input from user for num1 cout << "Enter the second number: "; cin>>num2;//get input from user for num2 cout << "Enter the third number: "; cin>>num3;//get input from user for num3 if(num1<=num2 && num1 <=num3){ cout<<"\n Smallest number is: "<<num1; }//num1 compare with num2 and num3 if(num2<=num1 && num2 <=num3){ cout<<"\n Smallest number is: "<<num2; }//num2 compare with num1 and num3 if(num3<=num1 && num3 <=num2){ cout<<"\n Smallest number is: "<<num3; }//num3 compare with num1 and num2 getch(); return 0; }
When the above code is compiled and executed, it produces the following results
Enter the first number: 54 Enter the second number: 23 Enter the third number: 32 Smallest number is: 23
Among float numbers
program 2
#include <iostream> #include <conio.h> using namespace std; int main() { double num1,num2,num3; //declare the variables cout << "Enter the first number: "; cin>>num1;//get input from user for num1 cout << "Enter the second number: "; cin>>num2;//get input from user for num2 cout << "Enter the third number: "; cin>>num3;//get input from user for num3 if(num1<=num2 && num1 <=num3){ cout<<"\n Smallest number is: "<<num1; }//num1 compare with num2 and num3 if(num2<=num1 && num2 <=num3){ cout<<"\n Smallest number is: "<<num2; }//num2 compare with num1 and num3 if(num3<=num1 && num3 <=num2){ cout<<"\n The smallest number is: "<<num3; }//num3 compare with num1 and num2 getch(); return 0; }
When the above code is compiled and executed, it produces the following results
Enter the first number: 45.3 Enter the second number: 12.7 Enter the third number: 65.3 The smallest number is 12.7
Similar post
C code to smallest among three numbers
C++ code to smallest among three numbers
Python code to smallest among three numbers
Java code to find smallest of three numbers using method
C code to smallest among three numbers using function
C++ code to smallest among three numbers using function
Python code to smallest among three numbers using function
Java code to find smallest of three numbers using ternary operator
C code to smallest among three numbers using ternary operator
C++ code to smallest among three numbers using ternary operator
Suggested for you
Addition of two numbers in Java
Addition of two numbers in C++
Addition of two numbers in Python
Addition of two numbers in PHP
Addition of two numbers in JavaScript
Sum of two numbers in Java using method
Sum of two numbers in C++ using function
Sum of two numbers in Python using function
C program to add two numbers without using arithmetic operators
C++ program to add two numbers without using arithmetic operators
Python program to add two numbers without using arithmetic operators
Data types in C++ language
Single dimension Array in C++ language
Data type in C++ programming language