Cpp program to calculate electricity bill
- Home
- Bill calculation
- Cpp program to calculate electricity bill
- On
- By
- 0 Comment
- Categories: Bill calculation, Calculations
Cpp program to calculate electricity bill
Cpp program to calculate electricity bill
In this tutorial, we will discuss the Cpp program to calculate electricity bill
In this post, we will learn how to calculate electricity bill using if condition in the C++ programming language
We can calculate monthly electric power usage in many ways.
In this tutorial, we will write two programs calculating electricity bill in C++ language.
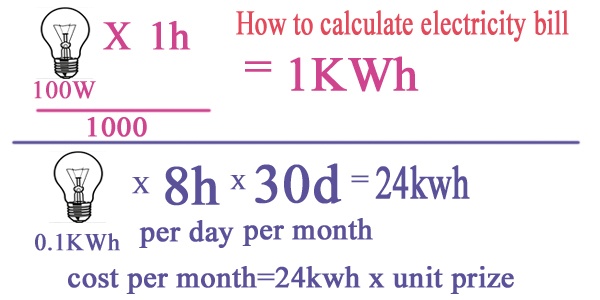
Before we write a program to calculate the electricity bill we must understand electricity charges and rates.
/*1 - 100 unit - 5/= 101-200 unit - 7/= 201-300 unit - 10/= above 300 - 15/=*/
This program can have the following common procedures for completion of this program
- Declare total unit consumed by the customer using the variable unit.
- If the unit consumed less or equal to 100 units, calculates the total amount of usage =unit*5
- If unit consumed between 100 to 200 units, calculates the total amount of usage =(100*5)+(unit-100)*7)
- If unit consumed between 200 to 300 units ,calculates total amount of usage =100*5)+(100*7)+(unit-200)*10
- If unit consumed above 300 units ,calculates total amount of usage =(100*5)+(100*7)+(100*10)+(unit-300)*15
- No additional charge
Electricity bill calculation – using normal method
program 1
#include <iostream> #include <conio.h> using namespace std; int main() { int unit=315; //declare variable unit //first we understand unit prize /*1 - 100 unit - 5/= 101-200 unit - 7/= 201-300 unit - 10/= above 300 - 15/=*/ if(unit<=100){//when this statement is true cout<<"Bill amount is: "; cout<<unit*5;//this statement is Executed otherwise } else if(unit<=200){//when this statement is true cout<<"Bill amount is: "; cout<<(100*5)+(unit-100)*7;//this statement is Executed otherwise } else if(unit<=300){//when this statement is true cout<<"Bill amount is: "; cout<<(100*5)+(100*7)+(unit-200)*10;//this statement is Executed otherwise } else if(unit>300){//when this statement is true cout<<"Bill amount is: "; cout<<(100*5)+(100*7)+(100*10)+(unit-300)*15;//this statement is Executed otherwise } else{//when all statements are false cout<<"Bill amount is: "; cout<<"No value";//this statement is Executed } getch(); return 0; }
When the above code is executed, it produces the following results
Bill amount is 2425
Electricity bill calculation – using take input from the user
Program 2
int main() { int unit; //declare variable unit //first we understand unit prize /*1 - 100 unit - 5/= 101-200 unit - 7/= 201-300 unit - 10/= above 300 - 15/=*/ cout<<"Enter the unit of usage: "; cin>>unit; if(unit<=100){//when this statement is true cout<<"Bill amount is: "; cout<<unit*5;//this statement is Executed otherwise } else if(unit<=200){//when this statement is true cout<<"Bill amount is: "; cout<<(100*5)+(unit-100)*7;//this statement is Executed otherwise } else if(unit<=300){//when this statement is true cout<<"Bill amount is: "; cout<<(100*5)+(100*7)+(unit-200)*10;//this statement is Executed otherwise } else if(unit>300){//when this statement is true cout<<"Bill amount is: "; cout<<(100*5)+(100*7)+(100*10)+(unit-300)*15;//this statement is Executed otherwise } else{//when all statements are false cout<<"Bill amount is: "; cout<<"No value";//this statement is Executed } getch(); return 0; }
When the above code is executed, it produces the following results
Enter the unit of usage: 350 Bill amount is: 2950
Electricity bill calculation – using & operator
program 3
#include <iostream> #include <conio.h> using namespace std; int main() { int unit; //declare variable unit //first we understand unit prize /*1 - 100 unit - 5/= 101-200 unit - 7/= 201-300 unit - 10/= above 300 - 15/=*/ cout<<"Enter the unit of usage"; cin>>unit; if(unit>0 && unit<=100){//when this statement is true cout<<"Bill amount is: "; cout<<unit*5;//this statement is Executed otherwise } else if(unit>100 && unit<=200){//when this statement is true cout<<"Bill amount is: "; cout<<(100*5)+(unit-100)*7;//this statement is Executed otherwise } else if( unit>200 && unit<=300){//when this statement is true cout<<"Bill amount is: "; cout<<(100*5)+(100*7)+(unit-200)*10;//this statement is Executed otherwise } else if(unit>300){//when this statement is true cout<<"Bill amount is: "; cout<<(100*5)+(100*7)+(100*10)+(unit-300)*15;//this statement is Executed otherwise } else{//when all statements are false cout<<"Bill amount is: "; cout<<"No value";//this statement is Executed } getch(); return 0; }
When the above code is executed, it produces the following results
Enter the unit of usage: 350 Bill amount is: 2950
Suggested for you
The data type in C++