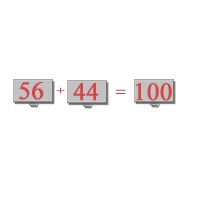
- On
- By
- 0 Comment
- Categories: addition, Calculations, function in C++
Cpp program to add two numbers using function
Cpp program to add two numbers using function
In this tutorial, we will discuss the Cpp program to add two numbers using the function.
In this topic, we will learn a simple concept of how to add two number(integer , floating point)using the function in the C++ programming language
already we will know the same concept using the operator.
if you known click here C++ program to sum of two numbers
This program has following procedures for completion
- function declaration or prototype
- variable declaration
- Read value from user
- function definition with return statements
- Call the function
- Display result on the screen
Cpp program to add two integer using function
Program 1
#include <iostream> #include <conio.h> using namespace std; int add(int x, int y);//function declaration or prototype int main() { int num1; //variable for store first numbers int num2; //variable for store second numbers int sum; //variable for store result of product //read numbers cout << "Enter the first number" << endl; cin>>num1; //stored first number- get input from user cout << "Enter the second number" << endl; cin>>num2; //stored second number- get input from user sum=add(num1, num2);//calling function and stored returning the result by function; //print sum of numbers cout<<"sum of two numbers "<<sum<<endl; getch(); return 0; } //function definition int add(int x, int y) { return (x+y); //return the product result to main }
When the above code is compiled and executed, it produces the following results
Enter the first number 23 Enter the second number 43 sum of two numbers 66
In the above program, deeply describe how to find sum of two integer using function in C++ language.
Cpp program to add two floating point numbers using function
Program 2
#include <iostream> #include <conio.h> using namespace std; float add(float x, float y);//function declaration or prototype int main() { float num1; //variable for store first number float num2; //variable for store second number float sum; //variable for store result of sum //read numbers cout << "Enter the first numbers" << endl; cin>>num1; //stored first number- get input from user cout << "Enter the second numbers" << endl; cin>>num2; //stored second number- get input from user sum=add(num1, num2);//calling function and stored returning the result by function; //print sum of numbers cout<<"Sum of two numbers "<<sum<<endl; getch(); return 0; } //function definition float add(float x, float y) { return (x+y); //return the addition result to main }
When the above code is compiled and executed, it produces the following results
Enter the first numbers 34.78 Enter the second number 45.34 Sum of two numbers 80.12
In the above program, deeply describe how to find sum of two floating point values using function in C++ language.
Similar post
Addition of two numbers in Java
Addition of two numbers in C++
Addition of two numbers in Python
Addition of two numbers in PHP
Addition of two numbers in JavaScript
Sum of two numbers in Java using method
Sum of two numbers in C++ using function
Sum of two numbers in Python using function
C program to add two numbers without using arithmetic operators
C++ program to add two numbers without using arithmetic operators
Python program to add two numbers without using arithmetic operators
Suggested for you
Data types in C++ language