C program triangle alphabet pattern using while loop
- Home
- Alphabet Pattern
- C program triangle alphabet pattern using while loop
- On
- By
- 0 Comment
- Categories: Alphabet Pattern, Floyd's triangle
C program triangle alphabet pattern using while loop
C program triangle alphabet pattern using while loop
In this tutorial, we will discuss C program triangle alphabet pattern using while loop
In this post, we will learn how to displayed Floyd’s triangle alphabet pattern using while loop or nested while loop in C programming language
here, we displayed some alphabet pyramid triangle program with coding using nested while loop and also we get input from user using scanf() function in C language
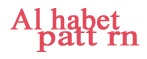
the user can provide numbers as they wish and get the alphabet pattern according to their input
Triangle alphabet pattern 1
Program 1
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: "); scanf("%d",&rows); i=1; while(i<=rows){ j=1; while(j<=i){ printf("%c",'A'-1+i); j++; } i++; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows: 6 A BB CCC DDDD EEEEE FFFFFF
Triangle alphabet pattern 2
Program 2
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: "); scanf("%d",&rows); i=1; while(i<=rows){ j=1; while(j<=i){ printf("%c",'A'-1+j); j++; } i++; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows: 6 A AB ABC ABCD ABCDE ABCDEF
Triangle alphabet pattern 3
Program 3
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: \n"); scanf("%d",&rows); i=1; while(i<=rows){ j=rows; while(j>=i) { printf("%C",'A'-1+i); j--; } i++; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows 6 AAAAAA BBBBB CCCC DDD EE F
Triangle alphabet pattern 4
Program 4
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: \n"); scanf("%d",&rows); i=rows; while(i>=1){ j=i; while(j<=rows) { printf("%c",'A'+j-1); j++; } i--; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows: 6 F EF DEF CDEF BCDEF ABCDEF
Triangle alphabet pattern 5
Program 5
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: \n"); scanf("%d",&rows); i=1; while(i<=rows){ j=i; while(j>=1) { printf("%c",'A'+j-1); j--; } i++; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows: 6 A BA CBA DCBA EDCBA FEDCBA
Triangle alphabet pattern 6
Program 6
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: "); scanf("%d",&rows); i=rows; printf("\n"); while(i>=1){ j=rows; while(j>=i) { printf("%c",'A'+j-1); j--; } i--; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter te number of rows: 6 F FE FED FEDC FEDCB FEDCBA
Triangle alphabet pattern 7
Program 7
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: "); scanf("%d",&rows); i=rows; printf("\n"); while(i>=1){ j=rows; while(j>=i) { printf("%c",'A'-1+i); j--; } i--; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows: 6 F EE DDD CCCC BBBBB AAAAAA
Triangle alphabet pattern 8
Program 8
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: "); scanf("%d",&rows); i=rows; printf("\n"); while(i>=1){ j=1; while(j<=i) { printf("%c",'A'-1+i); j++; } i--; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows: 6 FFFFFF EEEEE DDDD CCC BB A
Triangle alphabet pattern 9
Program 9
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: "); scanf("%d",&rows); printf("\n"); i=1; while(i<=rows){ j=rows; while(j>=i) { printf("%c",'A'-1+j); j--; } i++; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows: 6 FEDCBA FEDCB FEDC FED FE F
Triangle alphabet pattern 10
Program 10
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: "); scanf("%d",&rows); printf("\n"); i=rows; while(i>=1){ j=i; while(j>=1) { printf("%c",'A'-1+j); j--; } i--; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows: 6 FEDCBA EDCBA DCBA CBA BA A
Triangle alphabet pattern 11
Program 11
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; char ch='A'; printf("Enter the number of rows: \n"); scanf("%d",&rows); i=1; while(i<=rows){ j=1; while(j<=i){ printf("%c ",ch++); j++; } i++; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows 6 A B C D E F G H I J K L M N O P Q R S T U
Triangle alphabet pattern 12
Program 12
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows,num,count; printf("Enter the number of rows: \n"); scanf("%d",&rows); i=1; while(i<=rows){ num=rows-1; count=i; j=1; while( j<=i){ printf("%2c",(char)(count+64)); count=count+num; num--; j++; } i++; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows 6 A B G C H L D I M P E J N Q S F K O R T U
Triangle alphabet pattern 13
Program 13
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: \n"); scanf("%d",&rows); i=1; while(i<=rows){ j=1; while(j<=(i*2-1)){ printf("%c",(char)(j+64)); j++; } i++; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows 6 A ABC ABCDE ABCDEFG ABCDEFGHI ABCDEFGHIJK
Triangle alphabet pattern 14
Program 14
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows\n"); scanf("%d",&rows); i=1; while(i<=rows){ j=i; while(j<=rows){ printf("%c",(char)(j+64)); j++; } i++; printf("\n"); } getch(); return 0; }
When the above code executed, it produces the following results
Enter the number of rows 6 ABCDEF BCDEF CDEF DEF EF F
Similar post
Java program triangle alphabet pattern using while loop
C++ program triangle alphabet pattern using while loop
Java program to display Binary Pyramid pattern
C++ program to display Binary Pyramid pattern
C program to display hollow diamond star pattern
C++ program to display hollow diamond star pattern
Java code to print Rhombus and hollow Rhombus star pattern
C program to display Mirrored Rhombus hollow Mirrored Rhombus star pattern
C++ program to display Rhombus hollow Rhombus star pattern
Java program to display Parallelogram star pattern using while loop
C program to display Parallelogram star pattern using for loop
C++ program to display mirrored Parallelogram star pattern using for loop
Suggested for you
Nested for loop in Java language
Nested while loop in Java language
Data type in C++ language
Nested for loop in C++ language
Nested while loop in C++ language
Nested while loop in C language