C program to print Floyd’s triangle alphabet pattern
- Home
- Alphabet Pattern
- C program to print Floyd’s triangle alphabet pattern
- On
- By
- 0 Comment
- Categories: Alphabet Pattern, Floyd's triangle
C program to print Floyd’s triangle alphabet pattern
C program to print Floyd’s triangle alphabet pattern
In this tutorial, we will discuss the concept of C program to print Floyd’s triangle alphabet pattern
In this post, we will learn how to displayed Floyd’s triangle alphabet pattern using for loop or nested for loop in C programming language
here, we displayed 8 alphabet Floyd’s triangle program with coding using nested for loop and also we get input from user using scanf() function in C
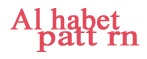
the user can provide numbers as they wish and get the alphabet pattern according to their input
Program 1
Floyd’s triangle alphabet pattern 1
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; char alphabet='A'; printf("Enter number of rows to pattern: "); scanf("%d",&rows); printf("\n"); for(i=1; i<=rows; i++){ for(j=1; j<=i; j++){ printf("%c",alphabet++); } printf("\n"); } printf("\nHere, your pattern is printed"); getch(); return 0; }
When the above code is executed, it produces the following results
Enter number of rows to pattern: 6 A BC DEF GHIJ KLMNO PORSTU Here, your pattern is printed
Program 2
Floyd’s triangle alphabet pattern 2
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows,num,count; char alphabet='A'; printf("Enter number of rows to pattern: "); scanf("%d",&rows); printf("\n"); for(i=1; i<=rows; i++){ num=rows-1; count=i; for(j=1; j<=i; j++){ printf("%2c",(char)(count+64)); count=count+num; num--; } printf("\n"); } printf("\nHere, your pattern is printed"); getch(); return 0; }
When the above code is executed, it produces the following results
Enter number of rows to pattern: 6 A B G C H L D I M P E J N Q S F K o R T U Here, your pattern is printrd
Program 3
Floyd’s triangle alphabet pattern 3
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows: "); scanf("%d",&rows); printf("\nyour pattern here\n\n"); for(i=0; i<=rows; i++){ for(j=i; j<=rows; j++){ printf("%c",(char)(j+65)); } printf("\n"); } getch(); return 0; }
When the above code is executed, it produces the following results
Enter the number of rows: 6 Your pattern here ABCDEFG BCDEFG CDEFG DEFG EFG FG G
Program 4
Floyd’s triangle alphabet pattern 4
#include <stdio.h> #include <stdlib.h> int main() { char web[]="Code4coding"; int i,j; for(i=0; web[i]; i++){ for(j=0; j<=i; j++){ printf("%c",web[j]); } printf("\n"); } return 0; }
When the above code is executed, it produces the following results
C Co Cod Code Code4 Code4c Code4co Code4cod Code4codi Code4codin Code4coding
Program 5
Floyd’s triangle alphabet pattern 5
#include <stdio.h> #include <stdlib.h> int main() { int i,j,k,rows; printf("Enter the number of rows: "); scanf("%d",&rows); printf("\nyour pattern here\n\n"); for(i=1; i<=rows; i++){ for(j=1; j<=rows-i; j++){ printf(" "); } for(k=1; k<=i; k++){ printf("%c",(char)(j+64)); } printf("\n"); } getch(); return 0; }
When the above code is executed, it produces the following results
Enter the number of rows: 6 your pattern here F EE DDD CCCC BBBBB AAAAAA
Program 6
Floyd’s triangle alphabet pattern 6
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows"); scanf("%d",&rows); for(i=1; i<=rows; i++){ for(j=1; j<=(i*2-1); j++){ printf("%c",(char)(j+64)); } printf("\n"); } printf("\nyour pattern here!\n"); return 0; }
When the above code is executed, it produces the following results
Enter the number of rows: 6 A ABC ABCDE ABCDEFG ABCDEFGHI ABCDEFGHIJK Your pattern here
Program 7
Floyd’s triangle alphabet pattern 7
#include <stdio.h> #include <stdlib.h> int main() { int i,j,rows; printf("Enter the number of rows"); scanf("%d",&rows); for(i=1; i<=rows; i++){ for(j=1; j<=(rows-i+1); j++){ printf("%c",(char)(j+64)); } printf("\n"); } printf("\nyour pattern here!\n"); getch(); return 0; }
When the above code is executed, it produces the following results
Enter the number of rows: 6 ABCDEF ABCDE ABCD ABC AB A your pattern here
Program 8
Floyd’s triangle alphabet pattern 8
#include <stdio.h> #include <stdlib.h> int main() { int i,j,k,rows; printf("Enter the number of rows: "); scanf("%d",&rows); for(i=rows; i>=1; i--){ k=i; for(j=1; j<=i; j++,k++){ printf("%c",(char)(k+64)); } printf("\n"); } printf("\nyour pattern here!\n"); getch(); return 0; }
When the above code is executed, it produces the following results
Enter the number of rows: 6 FGHIJK EFGHI DEFG CDE BC A Your pattern here
Suggested for you
Operator in C language
for loop in C language