C++ program: Find the frequency of each character in the string
- Home
- Find elements
- C++ program: Find the frequency of each character in the string
- On
- By
- 0 Comment
- Categories: Find elements, String
C++ program: Find the frequency of each character in the string
C++ program: Find the frequency of each character in the string
In this article, we will discuss the concept of the C++ program: Find the frequency of each character in the string
In this post, we are going to learn how to find the frequency of the each character of the string in C++ programming language
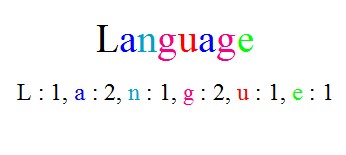
Code to find the frequency of the each character in the string
find the frequency of the each character in the string using for loop
The program allows the user to enter a String and then it finds the frequency of the each character in the string using for loop in C++ programing language
program 1
#include <iostream> #include <conio.h> #include <stdio.h> using namespace std; int main() { char str[100]; int i; int freq[256]={0}; cout<<"Enter a string\n"; gets(str); for(i=0; str[i]!='\0'; i++){ freq[str[i]]++; } for(i=0; i<256; i++){ if(freq[i] !=0){ cout<<"Character "<<(char)i<<" occurs "<<freq[i]<<" time in the string\n"; } } getch(); return 0; }
When the above code is executed,it produces the following result
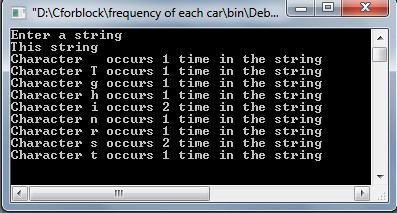
find the frequency of the each character in the string using while loop
The program allows the user to enter a String and then it finds the frequency of the each character in the string using while loop in C++ programing language
Program 2
#include <iostream> #include <conio.h> #include <stdio.h> using namespace std; int main() { char str[100]; int i; int freq[256]={0}; cout<<"Enter a string\n"; gets(str); i=0; while(str[i]!='\0'){ freq[str[i]]++; i++; } i=0; while(i<256){ if(freq[i] !=0){ cout<<"Character "<<(char)i<<" occurs "<<freq[i]<<" time in the string\n"; } i++; } getch(); return 0; }
When the above code is executed,it produces the following result
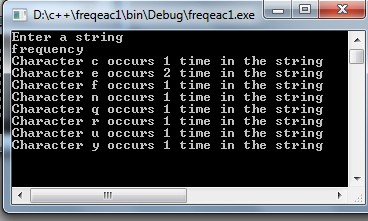
find the frequency of the each character in the string using do-while loop
The program allows the user to enter a String and then it finds the frequency of the each character in the string using do-while loop in C++ programing language
Program 3
#include <iostream> #include <conio.h> #include <stdio.h> using namespace std; int main() { char str[100]; int i; int freq[256]={0}; cout<<"Enter a string\n"; gets(str); i=0; do{ freq[str[i]]++; i++; } while(str[i]!='\0'); i=0; do{ if(freq[i] !=0){ cout<<"Character "<<(char)i<<" occurs "<<freq[i]<<" time in the string\n"; } i++; }while(i<256); getch(); return 0; }
When the above code is executed,it produces the following result
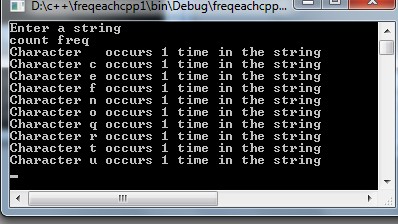
Suggested for you
Similar post
C++ program to count the total number of characters in the given string
C program to count the total number of characters in the given string
Python program to count the total number of characters in the given string
Java program to count the frequebcy of a character of a string
C program to count the frequebcy of a character of a string
Python program to count the frequebcy of a character of a string
C++ program to count the frequebcy of a character of a string