C++ program: find first n prime numbers
- Home
- Find elements
- C++ program: find first n prime numbers
- On
- By
- 0 Comment
- Categories: Find elements, Loop, prime
C++ program: find first n prime numbers
C++ program: find first n prime numbers
In this tutorial, we will discuss the concept of an Example C++ Program: find first n prime numbers in C++ language
In this post, we are going to learn how to write a program to find the first n prime number using for, while, and do-while loops in C++ programming language:
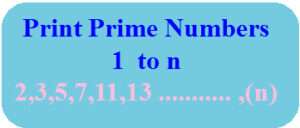
The number that can be divided by 1 and itself only. it is called the prime number
for Example 2,3,5,7,11,13, ….
To understand this example program, you should have previous knowledge of the following Java topics
Example program to print first n prime numbers
Print the first n prime number using the for loop
In this program, we will print the first “n” prime numbers, using for loop. First, the user is asked to enter a number randomly for n, and then the program prints the prime numbers 1 to n.
Program 1
//C++ program to find first n prime numbers #include <iostream> #include <conio.h> using namespace std; int main() { //declare and initialize the variable int n,i=3,count1,num; cout<<"Enter the count for n to print (0 -n)prime:\n"; //ask input from the user and store in n cin>>n; //Generating prime numbers if(n>=1){ cout<<"The First "<<n<<" prime numbers are: \n"; cout<<"2 "; } for(count1=2; count1<=n; i++){ for(num=2; num<i; num++){ if(i%num==0) break; } if(num==i){ cout<<i<<" "; count1++; } } getch(); return 0; }
When the above code is executed, it produces the following result
Enter the count for n to print (0 -n)prime: 6 The First 6 prime numbers are 2 3 5 7 11 13
Print the first n prime number using the while loop
In this program, we will print the first “n” prime numbers, using a while loop. first, the user is asked to enter a number randomly for n, and then the program prints the prime numbers 1 to n.
Program 2
//C++ program to find first n prime numbers #include <iostream> #include <conio.h> using namespace std; int main() { //declare and initialize the variable int n,i=3,count1,num; cout<<"Enter the count for n to print (0 -n)prime:\n"; //ask input from the user and store in n cin>>n; //Generating prime numbers if(n>=1){ cout<<"irst "<<n<<" prime numbers are: \n"; cout<<"2 "; } count1=2; while(count1<=n){ num=2; while(num<i){ if(i%num==0) break; num++; } if(num==i){ cout<<i<<" "; count1++; } i++; } getch(); return 0; }
When the above code is executed, it produces the following result
Enter the count for n to print (0 -n)prime: 15 The First 15 prime numbers are 2 3 5 7 11 13 17 19 23 29 31 37 41 43 47
Print the first n prime number using the do-while loop
In this program, we will print the first “n” prime numbers, using a do-while loop. first, the user is asked to enter a number randomly for n, and then the program prints the prime numbers 1 to n.
Program 3
//C++ program to find first n prime numbers #include <iostream> #include <conio.h> using namespace std; int main() { //declare and initialize the variable int n,i=3,count1,num; cout<<"Enter the count for n to print (0 -n)prime:\n"; //ask input from the user and store in n cin>>n; //Generating prime numbers if(n>=1){ cout<<"irst "<<n<<" prime numbers are: \n"; cout<<"2 "; } count1=2; do{ num=2; do{ if(i%num==0) break; num++; } while(num<i); if(num==i){ cout<<i<<" "; count1++; } i++; }while(count1<=n); getch(); return 0; }
When the above code is executed, it produces the following result
Enter the count for n to print (0 -n)prime:
10
The First 10 prime numbers are
2 3 5 7 11 13 17 19 23 29
Suggested for you
Similar post
Program to find whether a Number is Prime or Not in C++
Program to find whether a Number is Prime or Not in C
Program to find whether a Number is Prime or Not in Java
Program to find whether a Number is Prime or Not in Python
C++ program to find first n prime numbers
Java program to find first n prime numbers
C program to find first n prime numbers
C code to 5 ways to check whether the given integer is Even or Odd
Java code to 5 ways to check whether the given integer is Even or Odd
C++ code to 5 ways to check whether the given integer is Even or Odd
Python code to 5 ways to check whether the given integer is Even or Odd