C function to check a number is even or odd
- Home
- Check value
- C function to check a number is even or odd
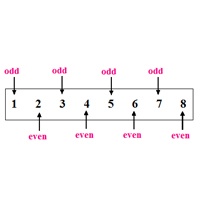
- On
- By
- 0 Comment
- Categories: Check value, Find elements
C function to check a number is even or odd
C function to check a number is even or odd
In this tutorial, we will discuss the C function to check a number is even or odd
In this program, we are going to learn about how to find odd or even number from given number using function in C language
First, we must understand what is even or odd?
What is Even or Odd
When the number is divided by 2 and the balance becomes zero. the above number is called as the even number – eg 2,4,6,8,10
and on the other sides when it is divided by 2 and balance becomes 1 they are called odd numbers or uneven numbers
In my previous post, I have explained the various ways to check whether the number is even or odd in C language
we can embed the logic of the program to check even or odd numbers using any of the following approaches in a function
here, I have explained how to embed in the function and how to check even number or odd number of the given number
Once This program received the number it will check the given number either odd or even using the function.
After receiving the input from the user, it is stored in the variable named num and then the program divides the value of num by 2 and displays the output
Check a number is even or odd using Modular operator
Program 1
#include <stdio.h> #include <stdlib.h> int find_Num(int);//function prototype int main() { int num; printf("Enter a number to check odd or even\n"); scanf("%d",&num); find_Num(num);//calling the function getch(); return 0; } //create function int find_Num(int num){//function definition if(num%2==0){ printf("\n%d is an even number",num); } else{ printf("\n%d is an odd number",num); } }
When the above code is executed, it produces the following results
Case 1
Enter a number to check odd or even 46 46 is an even number
Case 2
Enter a number to check odd or even 17 17 is an odd number
Program 2
Check a number is even or odd using modular with the return
#include <stdio.h> #include <stdlib.h> int find_Num(int);//function prototype int main() { int num; printf("Enter a number to check odd or even\n"); scanf("%d",&num); if(num%2==0){ printf("\n you entered number %d is a even number",num); } else{ printf("\nyou entered number %d is a odd number",num); } find_Num(num);//calling the function getch(); return 0; } //create function int find_Num(int num){//function definition return(num%2==0);//return value }
When the above code is executed, it produces the following results
Case 1
Enter a number to check odd or even 34 you entered number 34 is a even number
Case 2
Enter a number to check odd or even 45 you entered number 45 is a odd number
Check a number is even or odd using the function with conditional operator
#include <stdio.h> #include <stdlib.h> int oddEvenNum(int);//function prototype int main() { int num; printf("Enter an integer number: "); scanf("%d",&num); oddEvenNum(num);//calling the function getch(); return 0; } //create a function int oddEvenNum(int num){//function definition (num%2==0) ? printf("%d is an even",num): printf("%d is an odd",num); }
When the above code is executed, it produces the following results
Case 1
Enter an integer number 16 16 is an even
Case 2
Enter an integer number 57 57 is an odd
Check a number is even or odd using the function with Bitwise operator
#include <stdio.h> #include <stdlib.h> int oddEvenNum1(int);//function prototype int main() { int num; printf("Enter an integer number: "); scanf("%d",&num); oddEvenNum1(num);//calling the function getch(); return 0; } int oddEvenNum1(int num){//function definition if(num&1) printf("%d is an odd number",num); else printf("%d is an even number",num); }
When the above code is executed, it produces the following results
Case 1
Enter an integer number: 786 786 is an even number
Case 2
Enter an integer number: 89 89 is an odd number
Check a number is even or odd using the function with Division operator
#include <stdio.h> #include <stdlib.h> int find_Evenodd(int);//function prototype int main() { int num; printf("Enter a number for find odd or even\n"); scanf("%d",&num); find_Evenodd(num);//calling the function getch(); return 0; } //Create function int find_Evenodd(int num){//function definition if((num/2)*2==num) printf("%d is an even",num); else printf("%d is an odd",num); }
When the above code is executed, it produces the following results
Case 1
Enter a number for find odd or even 87 87 is an odd
Case 2
Enter a number for find odd or even 106 106 is an even
Check a number is even or odd using the function with Shift operator
#include <stdio.h> #include <stdlib.h> int find_Evenodd(int);//function prototype int main() { int num; printf("Enter a number for find even or odd\n"); scanf("%d",&num); find_Evenodd(num);//function call getch(); return 0; } int find_Evenodd(int num){//function definition if(((num>>1)<<1)==num ){ printf("%d is an even number ",num ); } else{ printf("%d is an odd number ",num ); } }
When the above code is executed, it produces the following results
Case 1
Enter a number for find even or odd 901 901 is an odd number
Case 2
Enter a number for find even or odd 1060 1060 is an even number
Similar post
C program to find a number is even or odd using the function
C program to separate Odd and Even numbers from an array
C program to Electricity bill calculation using the function
C program to display all even and odd numbers from 1 to n
C program display odd and even numbers without if statements
C program to calculate the sum of odd and even numbers
C program to find whether a number is even or odd
Suggested for you