- On
- By
- 0 Comment
- Categories: pointer, subtraction
C++ Example to subtract two integer using pointer
C++ Example to subtract two integer using pointer
In this article, we will discuss the concept of the C++ Example to subtract two integer using pointer in C++ programming language
In this post, we are going to learn how to write a program to find the subtraction of two numbers using pointer in C++ programming language
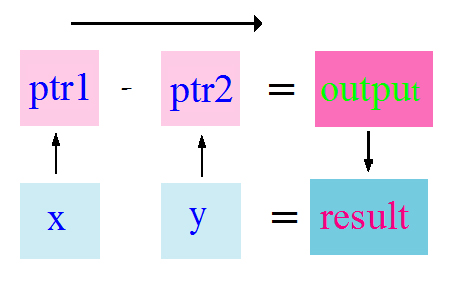
Code to find the subtraction of two numbers
Subtract two integer using pointer
The program use to calculate subtraction of given two integer numbers using pointer in C++ language
Program 1
#include <iostream> #include <conio.h> using namespace std; int main() { int num1,num2; //1 declare variable int *ptr1,*ptr2; //2 declare pointer variable int sub; //3 num1=3150; //4 variable initiakization num2=570; ptr1=&num1; ptr2=&num2; //5 assign the address of the variable to pointer variable sub=*ptr1 - *ptr2; //6 //calculate subtraction cout<<"Subtraction of the given two integer values is: "<<sub; //7 //find the output and display on the screen getch(); return 0; }
When the above code is executed, it produces the following result
Subtraction of the given two integer values is:2580
Subtract two integer using pointer – Takes input from the user
The program allow the user to enter two numbers and then calculates subtraction of given two integer numbers without using pointer in C++ language
Program 2
#include <iostream> #include <conio.h> using namespace std; int main() { int num1,num2; //1 int *ptr1,*ptr2; //2 int sub; //3 cout<<"Please enter value for num1: "; //4 cin>>num1; //5 cout<<"Please enter value for num2: "; //4 cin>>num2; //7 ptr1=&num1; //8 ptr2=&num2; sub=*ptr1 - *ptr2; //10 cout<<"Product of the two integer values is: "<<sub; //11 getch(); return 0; }
When the above code is executed, it produces the following result
Please enter value for num1:2000 Please enter value for num2: 500 Subtraction of the given two integer values is: 1500
Methods
- Create two variables to store two numbers as input provided by the user: num1,num2;
- Create two pointer variables (*ptr1,*ptr2)to store the address of the numbers: num 1 and num2
- Create a variable to store the subtraction of these numbers: sub
- Request the user to enter first input for store variable num1
- Using cin>> to store the first input value in num1
- Request the user to enter second input for store variable num2
- Using cin>> to store the second input value in num2
- Assign the address of variable num1 to ptr1
- Assign the address of variable num2 to ptr2
- Find the subtraction of two number using their addresses – find the sum of num1,num2 using their addresses and add them using the pointer variables
- Finally, display result on the screen- the subtraction of both numbers num1,num2
Similar post
C program find the sum of two numbers using the function
C program find the sum of two numbers using recursion
C program to find the product of two numbers using the pointer
C++ program find the sum of two numbers using the function
C++ program find the sum of two numbers using recursion
C++ program to find the product of two numbers using the pointer
Suggested for you
Data type in C++ language