C code to subtract two integer using Bitwise operator
- Home
- Calculations
- C code to subtract two integer using Bitwise operator
- On
- By
- 0 Comment
- Categories: Calculations, subtraction
C code to subtract two integer using Bitwise operator
C code to subtract two integer using Bitwise operator
In this article, we will discuss the concept of the C code to subtract two integer using Bitwise operator
In this post, we are going to learn how to write a program to find the subtraction of two numbers using Bitwise operator in C programming language
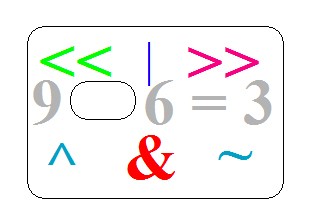
Code to find the subtraction of two numbers
Subtract two integer using Bitwise operator
The program allow the user to enter two integers and then calculates subtraction of given numbers using Bitwise operator in C language
Program 1
#include <stdio.h> #include <stdlib.h> int main() { int a,b,num1,num2;//variable declaration printf("Enter the first number: \n"); scanf("%d",&num1); //Ask input from the user and store the input in variable num1; printf("Enter the second number: \n"); scanf("%d",&num2); //Ask input from the user and store the input in variable num2; a=num1; //Assign the value of num1 to a b=num2; //Assign the value of num2 to b while (num2!= 0)//using while loop to calculate subtraction { int bwr=(~num1) & num2; num1=num1^num2; num2=bwr<<1; } printf("Subtraction of %d and %d is %d",a,b,num1); //display result on the screen getch(); return 0; }
When the above code is executed, it produces the following result
Case 1
Enter first number: 100 Enter second number: 55 Subtraction of two numbers 100 and 55 is: 45
Case 2
Enter first number: 55 Enter second number: 100 Subtraction of two numbers 55 and 100 is: -45
Subtract two integer using Bitwise operator – using function
The program allow the user to enter two integers and then calculates subtraction of given numbers using Bitwise operator in C language
Program 2
#include <stdio.h> #include <stdlib.h> int sub(int,int); int main() { int num1,num2,ans; printf("Enter the first number "); //ask input from the user scanf("%d",&num1); //store the input number in num1 printf("Enter the second number "); //ask input from the user scanf("%d",&num2); //store the input number in num1 ans=sub(num1,num2);//Calling the function printf("Subtraction of %d and %d is: %d",num1,num2,ans); getch(); return 0; } int sub(int x, int y){//function definition while(y!=0){ int carry=(~x) & y; x=x^y; y=carry<<1; } return x; }
When the above code is executed, it produces the following result
Case 1
Enter first number: 500 Enter second number: 230 Subtraction of two numbers 500 and 230 is: 270
Case 2
Enter first number: 200 Enter second number: 340 Subtraction of two numbers 50 and 23 is: -140
Suggested for you
Function in C language
Similar post
Subtract two numbers in C language
Subtract two numbers using function in C language
Subtract two numbers using recursion in C language
Find sum of two numbers in C language
Find sum of two numbers in C language using pointer
Find sum of two numbers in C language using function
Find sum of two numbers in C using recursion
Find sum of two numbers in C without Arithmetic operator
Find sum of natural numbers in C language
Find sum of natural numbers in C language using recursion