C++ code to subtract two integer using Bitwise operator
- Home
- Calculations
- C++ code to subtract two integer using Bitwise operator
- On
- By
- 0 Comment
- Categories: Calculations, subtraction
C++ code to subtract two integer using Bitwise operator
C++ code to subtract two integer using Bitwise operator
In this article, we will discuss the concept of the C++ code to subtract two integer using Bitwise operator
In this post, we are going to learn how to write a program to find the subtraction of two numbers using Bitwise operator in C++ programming language
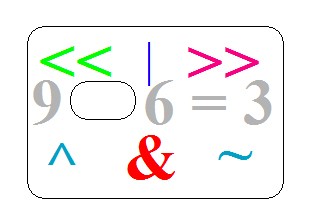
Code to find the subtraction of two numbers
Subtract two integer using Bitwise operator
The program allow the user to enter two integers and then calculates subtraction of given numbers using Bitwise operator in C++ language
Program 1
#include <iostream> #include <conio.h> using namespace std; int main() { int a,b,num1,num2; //variable declaration cout<<"Enter first number: \n"; //Ask input from the user cin>>num1; //Store the value in the num1 variable cout<<"Enter second number: \n"; //Ask input from the user cin>>num2; //Store the value in the num2 variable a=num1; //Assign the value of num1 to variable a b=num2; //Assign the value of num2 to variable b while (num2!= 0)//Calculate subtraction of the numbers using bitwise operator { int count=(~num1) & num2; num1=num1^num2; num2=count<<1; } cout<<"Subtraction of two numbers "<<a<<" and "<<b<<" is: "<<num1; //Display result on the screen getch(); return 0; }
When the above code is executed , it produces the following result
Case 1
Enter first number: 100 Enter second number: 45 Subtraction of two numbers 100 and 45 is: 55
Case 2
Enter first number: 20 Enter second number: 40 Subtraction of two numbers 20 and 40 is: -20
Subtract two integer using Bitwise operator-using function
The program allow the user to enter two integers and then calculates subtraction of given numbers using Bitwise operator in C++ language
Program 2
#include <iostream> #include <conio.h> using namespace std; int sub(int,int); int main() { int num1,num2,ans; cout<<"Enter two numbers: \n"; cin>>num1>>num2; ans=sub(num1,num2);//Calling the function cout<<"Subtraction of "<<num1<<" and "<<num2<<" is "<<ans; getch(); return 0; } int sub(int x, int y){//function definition while(y!=0){ int carry=(~x) & y; x=x^y; y=carry<<1; } return x; }
When the above code is executed , it produces the following result
Case 1
Enter two numbers: 1200 700 Subtraction of 1200 and 700 is: 500
Case 2
Enter two numbers: 700 1200 Subtraction of 700 and 1200 is: -500
Suggested for you
Data type in C++ language
Similar post
Subtract two numbers in C++ language
Subtract two numbers using function in C++ language
Subtract two numbers using recursion in C++ language
Find sum of two numbers in C++
Find sum of two numbers in C++ using recursion
Find sum of two numbers in C++ without Arithmetic operator